This blog describes How to Use Arrays in Go.
Arrays in Go are fixed-size collections of elements of the same type. The size of an array is part of its type, so they cannot be resized after they are declared. The following section describes how todeclare and access arrays.
Declaration
You can declare an array using the following syntax.
var arrayName [size]dataType
For example.
var numbers [5]int // Array of 5 integers
Initialization
Arrays can be initialized when declared or later using array literals.
var numbers = [5]int{1, 2, 3, 4, 5} // Initializing array when declared
// Later initialization
var numbers [5]int
numbers = [5]int{1, 2, 3, 4, 5}
Accessing Elements
You can access individual elements of an array using square brackets []
notation with the index of the element.
for i := 0; i < len(numbers); i++ {
fmt.Println(numbers[i])
}
Alternatively, you can use a range-based for
loop.
for index, value := range numbers {
fmt.Printf("numbers[%d] = %d\n", index, value)
}
Arrays are Value Types
In Go, when you assign one array to another, a copy of the original array is created.
a := [3]int{1, 2, 3}
b := a // b is a copy of a
Array Size
The size of an array is part of its type. Therefore, two arrays with different sizes are considered different types.
var arr1 [3]int
var arr2 [5]int
Arrays in Functions
Arrays can be passed to functions as arguments. However, since they are value types, passing a large array to a function can be inefficient. In practice, slices are often used instead for passing around collections of data. For example.
package main
import "fmt"
// Function to modify slice elements
func modifySlice(slice []int) {
slice[0] = 100
slice[1] = 200
slice[2] = 300
}
// Function to print slice elements
func printSlice(slice []int) {
for _, value := range slice {
fmt.Println(value)
}
}
func main() {
// Declare and initialize a slice
numbers := []int{1, 2, 3}
fmt.Println("Before modification:")
printSlice(numbers) // Print original slice
fmt.Println("After modification:")
modifySlice(numbers) // Modify slice
printSlice(numbers) // Print modified slice
}
Output

This covers the basics of arrays in Go. They provide a simple way to work with collections of data with a fixed size. However, slices, a more flexible data structure and they are more commonly used in Go for dynamic collections.
Further Reading
Variables and Data Types in Go
Go Programming Practice Exercise
Program Structure in Go Programming Language
Spring Framework Practice Problems and Their Solutions
How to Create and Run a Simple Program in Go?
20+ Interview Questions on Go Programming Language
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
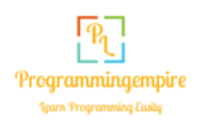