This blog describes Variables and Data Types in Go.
In Go, variables are statically typed, which means that the type of a variable is determined at compile time and cannot change during runtime. Here’s an overview of variables and data types in Go.
Basic Data Types
- Numeric Types
int
: Signed integer, size varies based on the platform (32 or 64 bits).int8
,int16
,int32
,int64
: Signed integers of specific sizes.uint
: Unsigned integer, size varies based on the platform.uint8
,uint16
,uint32
,uint64
: Unsigned integers of specific sizes.uintptr
: Unsigned integer type that is large enough to store the uninterpreted bits of a pointer value.float32
,float64
: Floating-point numbers.complex64
,complex128
: Complex numbers.
- Boolean Type
bool
: Represents true or false values.
- String Type
string
: Sequence of bytes, immutable.
Derived Data Types
- Array
array
: Fixed-size collection of elements of the same type. Arrays in Go are declared using[size]type
. The size of the array must be known at compile time.
- Slice
slice
: Dynamic and flexible version of arrays. Slices are like references to arrays and are declared using[]type
. They can grow or shrink as needed.
- Map
map
: Unordered collection of key-value pairs. Maps are declared usingmap[keyType]valueType
.
- Struct
struct
: Composite data type that groups together variables of different types under a single name. Structs are declared using thetype
andstruct
keywords.
- Pointer
pointer
: Variables that store the memory address of another variable. Pointers are declared using the*
symbol followed by the type of the variable it points to.
Type Conversion
Go requires explicit type conversion when converting between types. Type conversion is done using syntax like typeName(expression)
.
Zero Values
Variables in Go are automatically assigned a “zero value” if not explicitly initialized. Zero values depend on the type of the variable. For example, the zero value of an int
is 0
, and the zero value of a string
is an empty string ""
.
Constants
Constants are like variables, but their values cannot be changed after they are declared. Constants are declared using the const
keyword.
Example
package main
import "fmt"
func main() {
// Numeric types
var i int = 42
var f float64 = 3.14
var b bool = true
var s string = "Hello, Go!"
// Derived types
var arr [3]int = [3]int{1, 2, 3}
var slice []int = []int{4, 5, 6}
var m map[string]int = map[string]int{"a": 1, "b": 2}
type Person struct {
Name string
Age int
}
var p Person = Person{Name: "Alice", Age: 30}
var ptr *int = &i
// Printing values
fmt.Println(i, f, b, s)
fmt.Println(arr, slice, m, p, *ptr)
}
Output
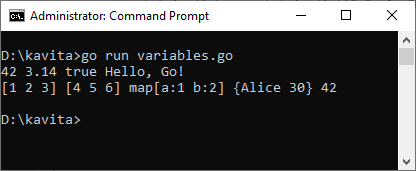
Further Reading
Program Structure in Go Programming Language
Spring Framework Practice Problems and Their Solutions
How to Create and Run a Simple Program in Go?
20+ Interview Questions on Go Programming Language
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
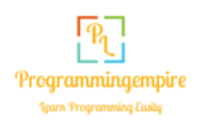