This blog describes the Program Structure in Go Programming Language.
In Go, program structure follows a straightforward approach. Here’s a basic breakdown.
Package Declaration
Every Go file starts with a package declaration. This declaration indicates the package to which the file belongs. Packages are Go’s way of organizing and reusing code. For example.
package main
Import Statements
After the package declaration, you import other packages that your program will use. Go provides a rich standard library, and you can also import third-party packages. For example.
import "fmt"
Main Function
The main
function is the entry point of the executable programs in Go. It is where the execution of the program begins. It must be in the main
package. For example.
func main() {
// Your code here
}
Other Functions and Declarations
After the main
function, you can define other functions, types, constants, and variables that your program needs. For example.
func add(a, b int) int {
return a + b
}
Comments
Go supports both single-line and multi-line comments. Comments are crucial for documenting your code and making it understandable for others. For example.
// This is a single-line comment
/*
This is a multi-line comment
*/
Variables and Constants
Go is a statically typed language, meaning you have to declare the type of variables. You can use the var
keyword for variables and const
for constants. For example.
var x int = 5
const y = 10
Control Structures
Go has typical control structures like if statements, for loops, and switch cases. For example.
if x > 0 {
fmt.Println("x is positive")
} else {
fmt.Println("x is non-positive")
}
for i := 0; i < 5; i++ {
fmt.Println(i)
}
switch x {
case 1:
fmt.Println("One")
case 2:
fmt.Println("Two")
default:
fmt.Println("Other")
}
Error Handling
Go emphasizes error handling through its built-in error type and the error
interface. Functions can return multiple values, with the last one typically being an error. For example.
result, err := someFunction()
if err != nil {
fmt.Println("Error:", err)
return
}
fmt.Println(result)
Concurrency
Go has strong support for concurrency through goroutines and channels. Goroutines are lightweight threads managed by the Go runtime, and channels are used for communication between them. For example.
func main() {
ch := make(chan int)
go func() {
ch <- 42
}()
fmt.Println(<-ch) // Prints 42
}
Defer, Panic, and Recover
Go provides mechanisms for handling exceptional situations. defer
schedules a function call to be run after the function completes. panic
is used to abort a function execution due to a runtime error. recover
is used to regain control of a panicking goroutine. For example.
func main() {
defer fmt.Println("deferred")
panic("panic occurred")
}
These elements make up the basic structure of a Go program. As you become more familiar with the language, you’ll start to appreciate its simplicity and efficiency in expressing complex ideas.
Further Reading
Spring Framework Practice Problems and Their Solutions
How to Create and Run a Simple Program in Go?
20+ Interview Questions on Go Programming Language
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
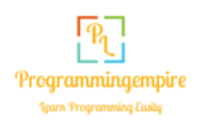