In this blog 20 Go Programming Practice Problems are provided. Also, their solutions are provided.
The following list contains 20 practice problems to help you improve your skills in Go programming.
- Write a program to find the sum of all elements in an integer array.
- Implement a function to check if a given string is a palindrome.
- Write a program to find the factorial of a given number.
- Implement a function to check if a given number is prime.
- Write a program to find the Fibonacci sequence up to a specified number of terms.
- Implement a function to reverse a string.
- Write a program to find the maximum and minimum elements in an integer array.
- Implement a function to check if a given integer is a perfect square.
- Write a program to count the number of vowels and consonants in a given string.
- Implement a function to find the GCD (Greatest Common Divisor) of two integers.
- Write a program to find the intersection of two integer arrays.
- Implement a function to convert a decimal number to binary.
- Write a program to sort an integer array in ascending order using the bubble sort algorithm.
- Implement a function to find the average of elements in an integer array.
- Write a program to check if a given year is a leap year.
- Implement a function to find the median of elements in an integer array.
- Write a program to check if a given string is an anagram of another string.
- Implement a function to find the square root of a given number using the Newton-Raphson method.
- Write a program to remove duplicate elements from an integer array.
- Implement a function to find the area of a triangle given the lengths of its sides.
These practice problems cover a range of topics and concepts in Go programming and can help you reinforce your understanding of the language. Happy coding!
Further Reading
Features of Go Programming Language
Benefits of Go Programming Language
Variables and Data Types in Go
Go Programming Practice Exercise
Applications of Go Programming Language
Program Structure in Go Programming Language
Spring Framework Practice Problems and Their Solutions
How to Create and Run a Simple Program in Go?
20+ Interview Questions on Go Programming Language
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
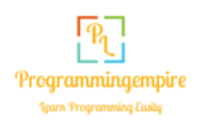