Here is the course outline for Building Dynamic Web Applications with the MERN Stack.
Dive into the world of full-stack web development with our project-based short-term course on the MERN (MongoDB, Express.js, React.js, Node.js) stack. The MERN stack is a popular choice for building modern, dynamic web applications, offering a powerful combination of technologies for both front-end and back-end development. Whether you’re a beginner or an experienced developer looking to expand your skill set, this course will provide you with the hands-on experience and practical knowledge needed to develop robust web applications using the MERN stack.
Course Outline
- Introduction to the MERN Stack
- Overview of MongoDB, Express.js, React.js, and Node.js
- Understanding the advantages of using the MERN stack
- Setting up the development environment
- Building a RESTful API with Node.js and Express.js
- Creating routes for CRUD operations
- Connecting to MongoDB database using Mongoose
- Handling middleware for authentication and validation
- Front-end Development with React.js
- Introduction to React components and JSX
- Managing state and props in React
- Building reusable UI components
- Integrating the Frontend with the Backend
- Making HTTP requests from React to the Express.js backend
- Consuming API endpoints in React components
- Handling asynchronous operations with Promises and async/await
- Managing State with Redux
- Understanding the Redux architecture and principles
- Setting up Redux store, actions, and reducers
- Connecting Redux with React components
- Authentication and Authorization
- Implementing user authentication using JSON Web Tokens (JWT)
- Securing routes and resources based on user roles
- Persisting user sessions and tokens
- Real-time Communication with WebSockets (Optional)
- Introduction to WebSockets and socket.io
- Implementing real-time chat or notifications in the application
- Deployment and Continuous Integration/Continuous Deployment (CI/CD)
- Deploying the application to cloud platforms like Heroku or AWS
- Setting up CI/CD pipelines for automated testing and deployment
- Project Work
- Working on a comprehensive project throughout the course
- Implementing features such as user authentication, CRUD operations, real-time updates, etc.
- Applying best practices for code organization, documentation, and scalability
Duration: 6-8 weeks (depending on intensity and frequency of classes)
Target Audience
- Web developers interested in mastering the MERN stack for building dynamic web applications.
- Front-end developers looking to transition into full-stack development.
- Students and professionals seeking practical experience in building real-world projects with modern web technologies.
Prerequisites
- Basic knowledge of HTML, CSS, and JavaScript.
- Familiarity with any programming language or framework is helpful but not required.
Delivery Method
- Instructor-led lectures and live coding sessions.
- Hands-on workshops and guided project development.
- Code reviews and feedback sessions.
- Access to online resources, documentation, and community support for further learning.
Upon completion of the course, participants will have the skills and confidence to develop full-stack web applications using the MERN stack, along with a solid understanding of best practices for building scalable, maintainable, and secure applications.
Mail us for queries: emailtoprogrammingempire@gmail.com
Further Reading
How to Master Full Stack Development?
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
100+ MCQs On Java Architecture
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
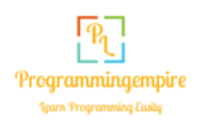