The following example code demonstrates how to write a Program to Upload a File in JSP.
Here is an example code for a JSP program that uploads a file.
- The form for file upload
<form action="upload" method="post" enctype="multipart/form-data">
<input type="file" name="file">
<input type="submit" value="Upload">
</form>
- The JSP page to handle the file upload
<%@ page import="java.io.*" %>
<%@ page import="javax.servlet.http.*" %>
<%
String savePath = application.getRealPath("/") + "uploads";
File fileSaveDir = new File(savePath);
if (!fileSaveDir.exists()) {
fileSaveDir.mkdir();
}
try {
Part part = request.getPart("file");
String fileName = extractFileName(part);
part.write(savePath + File.separator + fileName);
} catch (Exception e) {
out.println("File upload failed");
}
%>
<%!
private String extractFileName(Part part) {
String contentDisp = part.getHeader("content-disposition");
String[] items = contentDisp.split(";");
for (String s : items) {
if (s.trim().startsWith("filename")) {
return s.substring(s.indexOf("=") + 2, s.length() - 1);
}
}
return "";
}
%>
In this code, the form with the file input is used to submit the file to the JSP page, which handles the file upload. The file is saved in the uploads
directory under the web application’s root directory. The request.getPart()
method is used to retrieve the file being uploaded. The part.write()
method is used to write the file to disk. The extractFileName()
helper method is used to extract the original file name from the request header.
Further Reading
Understanding Enterprise Java Beans
- Android
- Angular
- ASP.NET
- AWS
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Deep Learning
- Django
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- Machine Learning
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- Solidity
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
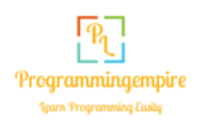