The following example code demonstrates how to write a Cookie Handling in JSP.
Here is an example code for a JSP program to create, read, and delete cookies.
- Create Cookie
<%
Cookie cookie = new Cookie("user", "John Doe");
cookie.setMaxAge(60 * 60 * 24 * 365); // 1 year
response.addCookie(cookie);
%>
2. Read Cookie
<%
Cookie[] cookies = request.getCookies();
if (cookies != null) {
for (Cookie cookie : cookies) {
if (cookie.getName().equals("user")) {
out.println("Cookie value: " + cookie.getValue());
}
}
}
%>
3. Delete Cookie:
<%
Cookie[] cookies = request.getCookies();
if (cookies != null) {
for (Cookie cookie : cookies) {
if (cookie.getName().equals("user")) {
cookie.setMaxAge(0);
response.addCookie(cookie);
}
}
}
%>
In this code, to create a cookie, a Cookie
object is created with a name and value, and the maximum age of the cookie is set to 1 year. The cookie is then added to the response using the response.addCookie()
method.
To read a cookie, the request.getCookies()
method is used to retrieve all cookies associated with the request. The cookies are then looped through, and if a cookie with the name “user” is found, its value is printed to the page using the out.println()
method.
To delete a cookie, the steps are similar to reading a cookie, except that the maximum age of the cookie is set to 0. This effectively deletes the cookie, as it will no longer be returned in subsequent requests.
Further Reading
Understanding Enterprise Java Beans
- Android
- Angular
- ASP.NET
- AWS
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Deep Learning
- Django
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- Machine Learning
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- Solidity
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
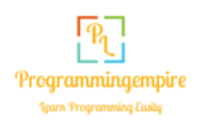