The following program demonstrates how to Print the ASCII value of Each Element in an Array in Java.
In this program, first, we initialize an integer array with numbers ranging from 0 to 255. Since char is an integer type in Java. Therefore, we can store characters in integer variables. When we typecast an integer into a char type, we get its equivalent ASCII character. So, here we use a for loop to iterate over each element of the array. Within the loop, we print each element by typecasting it to a char value. So, its ASCII value gets printed.
public class Exercise4
{
public static void main(String[] args) {
int[] myarray={80, 95, 121, 101, 45, 34, 66, 29, 82, 150, 61, 48, 12};
System.out.println("Array Elements with ASCII values...");
for(int i=0;i<myarray.length;i++)
{
System.out.print(myarray[i]+"\t");
}
System.out.println("\nArray Elements with Corresponding Character values...");
for(int i=0;i<myarray.length;i++)
{
System.out.print((char)myarray[i]+"\t");
}
}
}
Output

Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
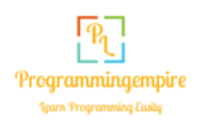