The following article demonstrates the Multiplication of Two Matrices in PHP.
In order to find the product of two matrices in PHP, you need to perform matrix multiplication using nested loops. The following code demonstrates the implementation.
// Define two matrices
$matrix1 = array(
array(1, 2, 3),
array(4, 5, 6),
);
$matrix2 = array(
array(7, 8),
array(9, 10),
array(11, 12),
);
// Get the dimensions of the matrices
$rows1 = count($matrix1);
$cols1 = count($matrix1[0]);
$rows2 = count($matrix2);
$cols2 = count($matrix2[0]);
// Initialize a result matrix with zeros
$result = array();
for ($i = 0; $i < $rows1; $i++) {
for ($j = 0; $j < $cols2; $j++) {
$result[$i][$j] = 0;
}
}
// Perform matrix multiplication
for ($i = 0; $i < $rows1; $i++) {
for ($j = 0; $j < $cols2; $j++) {
for ($k = 0; $k < $cols1; $k++) {
$result[$i][$j] += $matrix1[$i][$k] * $matrix2[$k][$j];
}
}
}
// Print the result matrix
echo "<table>";
foreach ($result as $row) {
echo "<tr>";
foreach ($row as $value) {
echo "<td>" . $value . "</td>";
}
echo "</tr>";
}
echo "</table>";
In this example, we define two matrices $matrix1
and $matrix2
with dimensions (2,3) and (3,2) respectively. We get the number of rows and columns of the matrices using the count()
function. Also, we initialize an empty array $result to store the result matrix and initialize it with zeros.
Then, we perform matrix multiplication using three nested loops and store the result in the $result
matrix. So, the outermost loop iterates for all rows of the first matrix, the innermost loop iterates for all the columns of the second matrix, and the middle loop iterates for the columns of the first matrix and the rows of the second matrix. Finally, we print the result matrix in tabular format using nested loops. The output of the above code will be as follows.
58 64
139 154
Also, note that the values in the result matrix are the product of the corresponding elements of the input matrices, computed using matrix multiplication.
Further Reading
Examples of Array Functions in PHP
Exploring PHP Arrays: Tips and Tricks
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
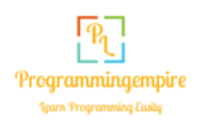