In this article, I will explain the concept of Method Overloading and Method Overriding in Java.
- Method overloading is when a class has multiple methods with the same name but with different parameter lists. Method overloading allows a class to have multiple methods with the same name that perform different tasks based on the parameters passed to the method.
- Method overriding is when a subclass provides a new implementation for a method that is already defined in its superclass. Method overriding allows a subclass to inherit a method from its superclass and to provide its own implementation for that method.
Example of Method Overloading in Java
class OverloadingExample {
public void display(int num) {
System.out.println("int: " + num);
}
public void display(float num) {
System.out.println("float: " + num);
}
public void display(double num) {
System.out.println("double: " + num);
}
}
Method Overriding in Java
class Shape {
public void draw() {
System.out.println("Drawing Shape");
}
}
class Circle extends Shape {
@Override
public void draw() {
System.out.println("Drawing Circle");
}
}
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
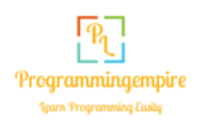