The following article demonstrates how to use JavaBean in JSP.
In order to create a JSP page that uses a Java Bean, follow these steps.
- Create a Java Bean class with properties and getter/setter methods for those properties. For example, consider a Java Bean class named Employee with name, age, and salary properties.
public class Employee {
private String name;
privateint age;
private double salary;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
publicintgetAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
}
2. After that, create a JSP page that uses Java Bean. In the JSP page, use the jsp:useBean tag to instantiate the Java Bean class, and use the jsp:setProperty tag to set the properties of the Java Bean. For example.
<jsp:useBean id="employee" class="com.example.Employee" />
<jsp:setProperty name="employee" property="name" value="John" />
<jsp:setProperty name="employee" property="age" value="30" />
<jsp:setProperty name="employee" property="salary" value="50000.00" />
<p>Name: ${employee.name}</p>
<p>Age: ${employee.age}</p>
<p>Salary: ${employee.salary}</p>
3. Finally, deploy and run the JSP page in a web container. When the JSP page is accessed, the Java Bean is instantiated and its properties are set using the jsp:setProperty tags. The values of the properties are then displayed using EL expressions.
So, by following these steps, you can create a JSP page that uses a Java Bean to store and display data.
Further Reading
Spring Framework Practice Problems and Their Solutions
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
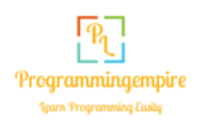