In this article on Java Strings – String, StringBuilder, and StringBuffer, I will explain the difference between these three string classes.
String
is an immutable class in Java that represents a sequence of characters. Once aString
object is created, its value cannot be changed.StringBuilder
andStringBuffer
are mutable classes in Java that represent a sequence of characters. The difference betweenStringBuilder
andStringBuffer
is thatStringBuilder
is not thread-safe, whileStringBuffer
is thread-safe.
Example of Creating Java Strings Using the String Class
String str1 = new String("Hello");
String str2 = "World";
String str3 = new String(new char[]{'H', 'i'});
Creating Java Strings Using the StringBuffer Class
StringBuffer sb1 = new StringBuffer("Hello");
StringBuffer sb2 = new StringBuffer(5);
sb2.append("World");
Using the StringBuilder Class to Create Java Strings
StringBuilder sb1 = new StringBuilder("Hello");
StringBuilder sb2 = new StringBuilder(5);
sb2.append("World");
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
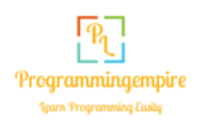