The following code shows an example of Inserting Record Using Prepared Statement in PHP.
Here, we use the prepare() function of the mysqli class. The PHP script in the code collects the data from the POST variables. In order to insert a record, the insert command uses the placeholders with ? symbol in place of the actual values. When the bind_param() function executes, it replaces the placeholders with the actual values. The execute() function executes the query. The first parameter of the bind function is a string containing as many characters as the number of parameters required by the insert command.
As can be seen the string “isii” indicates that the first parameter is an integer. Similarly, the rest of the three parameters are string, integer, and integer respectively.
Program for Inserting Record Using Prepared Statement in PHP
<html>
<head>
<title>Inserting Data</title>
</head>
<body>
<form name="f1" method="post" action="<?php echo $_SERVER['PHP_SELF']; ?>">
<table border="0">
<caption>Insert a Course Record</caption>
<tr>
<td>Enter Course Code: </td>
<td><input type="text" name="c1" /></td>
</tr>
<tr>
<td>Enter Course Name: </td>
<td><input type="text" name="c2" /></td>
</tr>
<tr>
<td>Enter Duration: </td>
<td><input type="text" name="c3" /></td>
</tr>
<tr>
<td>Enter Number of Credits: </td>
<td><input type="text" name="c4" /></td>
</tr>
<tr>
<td><input type="submit" name="submit" value="Insert"/></td>
</tr>
</table>
</form>
</body>
</html>
</html>
<?php
if(isset($_POST['submit'])){
$a=$_POST['c1'];
$b=$_POST['c2'];
$c=$_POST['c3'];
$d=$_POST['c4'];
$server_host="localhost";
$database_user="root";
$password="";
// Connect with MySQL Server
$con1=new mysqli($server_host, $database_user, $password, "institute");
// Create a query for database creation
$query="insert into course(course_id, course_name, duration, credits)values(?,?,?,?)";
$s=$con1->prepare($query);
$s->bind_param("isii", $x, $y, $z, $w);
$x=$a;
$y=$b;
$z=$c;
$w=$d;
$s->execute();
echo 'Record Inserted Successfully!';
$s->close();
$con1->close();
}
?>
Output
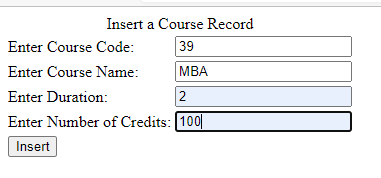
When we run the above program, it displays the above HTML form.

After providing the input values user clicks on the insert button. So, the PHP script executes and the record is inserted.
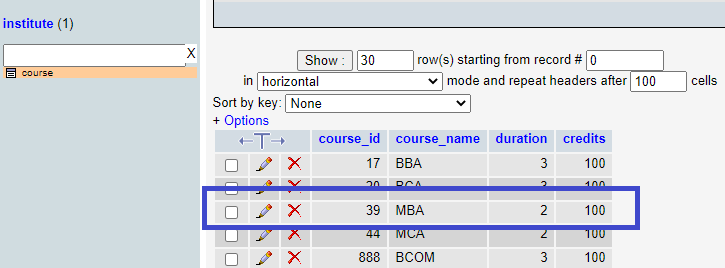
When you click on the course table in phpMyAdmin, the database table shown above indicates that the record is inserted.
Similarly, we can insert multiple records using a loop. The following example demonstrates it.
Likewise, we can also use the prepared statement in the select query. The following example demonstrates the select query using the prepared statement.
Further Reading
Examples of Array Functions in PHP