This article describes Inheritance and Polymorphism in Java.
- Basically, Inheritance is a mechanism in object-oriented programming that allows a subclass to inherit the properties and behaviors of its superclass. This allows for code reuse and the creation of a class hierarchy.
- In contrast, Polymorphism is a mechanism in object-oriented programming that allows an object to behave as an instance of its class or any of its super classes. As a result, method calls are dynamic. So, the program calls the methods on the basis of the actual type of object. Rather than according to their declared types.
Examples of Using Inheritance and Polymorphism in Java
As a matter of fact, inheritance enables a subclass to inherit the properties and methods of the super class. The following program demonstrates the use of inheritance in Java.
class Animal {
public void eat() {
System.out.println("Animal is eating");
}
}
class Dog extends Animal {
public void bark() {
System.out.println("Dog is barking");
}
}
public class Main {
public static void main(String[] args) {
Dog d = new Dog();
d.eat();
d.bark();
}
}
As can be seen, in this example, the Dog
class inherits from the Animal
class using the extends
keyword. Therefore, the Dog
class now has all the methods and fields of the Animal
class. Furthermore, it can also define its own methods and fields. Finally, the main
method creates a Dog
object. After that, its eat
and bark
methods are called. The following output appears.
Animal is eating
Dog is barking
Polymorphism in Java
The following program demonstrates the use of polymorphism in java.
class Animal {
public void makeSound() {
System.out.println("Animal sound");
}
}
class Dog extends Animal {
@Override
public void makeSound() {
System.out.println("Bark");
}
}
class Cat extends Animal {
@Override
public void makeSound() {
System.out.println("Meow");
}
}
public class Main {
public static void main(String[] args) {
Animal animal = new Animal();
Animal dog = new Dog();
Animal cat = new Cat();
animal.makeSound();
dog.makeSound();
cat.makeSound();
}
}
In this example, the Animal
, Dog
, and Cat
classes each have a method named makeSound
. This is an example of polymorphism because a single method name (makeSound
) is used to refer to different implementations in different classes. The program creates Animal objects in the main
method, Animal
objects for each of the three classes. Afterwards, their makeSound
methods are called. The following output appears.
Animal sound
Bark
Meow
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
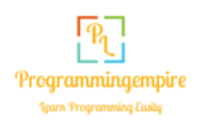