The following example code demonstrates How to Use forward() Method in a Servlet.
Here is an example of a dynamic web application using servlets to demonstrate the forward()
method.
import java.io.*;
import javax.servlet.*;
import javax.servlet.http.*;
public class ForwardServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
RequestDispatcher dispatcher = request.getRequestDispatcher("/ForwardedServlet");
dispatcher.forward(request, response);
}
}
import java.io.*;
import javax.servlet.*;
import javax.servlet.http.*;
public class ForwardedServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html>");
out.println("<head>");
out.println("<title>Forwarded Servlet</title>");
out.println("</head>");
out.println("<body>");
out.println("<h1>Forwarded Servlet</h1>");
out.println("<p>This page was generated by a servlet that was forwarded by another servlet.</p>");
out.println("</body>");
out.println("</html>");
}
}
In this example, the ForwardServlet
class handles GET requests and uses the forward()
method to forward the request to the ForwardedServlet
. The ForwardedServlet
generates an HTML response that is sent to the client. The forward()
method allows one servlet to delegate its processing to another servlet. This can be useful in situations where one servlet performs some initial processing and then delegates the remainder of the processing to another servlet. The forward()
method ensures that the response from the second servlet is returned to the client as if it had come directly from the first servlet.
Further Reading
Understanding Enterprise Java Beans
- Android
- Angular
- ASP.NET
- AWS
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Deep Learning
- Django
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- Machine Learning
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- Solidity
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
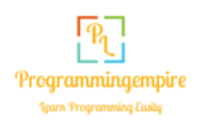