The following example code demonstrates How to Use an Iterator on an ArrayList in Java.
Problem Definition
In order to create an ArrayList of Companies, create a Company class that contains the company_id, company_name, and an ArrayList of Emp objects as its attributes. Display the details of companies using an iterator.
Here’s an example of how to create an ArrayList
of Company
objects and display the details of each company using an Iterator
.
import java.util.*;
class Employee {
private int id;
private String name;
private int age;
public Employee(int id, String name, int age) {
this.id = id;
this.name = name;
this.age = age;
}
public String toString() {
return "Employee [id=" + id + ", name=" + name + ", age=" + age + "]";
}
}
class Company {
private int id;
private String name;
private List<Employee> employees;
public Company(int id, String name) {
this.id = id;
this.name = name;
employees = new ArrayList<>();
}
public void addEmployee(Employee employee) {
employees.add(employee);
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("Company [id=" + id + ", name=" + name + "]\n");
for (Employee employee : employees) {
sb.append(" " + employee + "\n");
}
return sb.toString();
}
}
public class Main {
public static void main(String[] args) {
List<Company> companies = new ArrayList<>();
companies.add(new Company(1, "Google"));
companies.add(new Company(2, "Apple"));
companies.add(new Company(3, "Microsoft"));
companies.get(0).addEmployee(new Employee(101, "John Doe", 30));
companies.get(0).addEmployee(new Employee(102, "Jane Doe", 25));
companies.get(1).addEmployee(new Employee(103, "Jim Smith", 35));
companies.get(2).addEmployee(new Employee(104, "John Smith", 40));
companies.get(2).addEmployee(new Employee(105, "Jane Smith", 45));
System.out.println("Using Iterator:");
Iterator<Company> companyIt = companies.iterator();
while (companyIt.hasNext()) {
System.out.println(companyIt.next());
}
}
}
In this example, the Company
class has three attributes: id
, name
, and an ArrayList
of Employee
objects. The Company
class has a constructor that initializes the id
and name
attributes and creates a new ArrayList
of Employee
objects. The addEmployee
method is used to add Employee
objects to the employees
list. The toString
method is overridden to display the details of the company and its employees.
The ArrayList
of Company
objects is created in the main
method and Employee
objects are added to each company. The Iterator
is then used to traverse the companies
list and display the details of each company. The hasNext
method is used to check if there are more elements in the list
Further Reading
Understanding Enterprise Java Beans
- Android
- Angular
- ASP.NET
- AWS
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Deep Learning
- Django
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- Machine Learning
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- Solidity
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
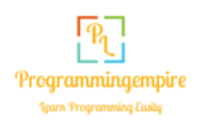