The following code explains How to Perform Recursion in C.
Basically, recursion refers to a function calling itself until a condition is met. Therefore, the terminating condition is very important for a recursive function. Otherwise, it results in an infinite number of function calls.
While, the first function computes the factorial of a number. So, when number becomes 0, it returns 1. Also, all previous calls to this function returned and the value n*(n-1)*(n-2)*……*1 is computed.
Similarly, the findsum() function returns when the number n becomes 0. Hence, this function computes n+(n-1)+(n-2)+…..+0 across all recursive calls.
Likewise, the function findpower() computes a raise to the power b for two integers a and b recursively. It computes a*a*…..*a*1 till be becomes 0 in the final recursive call.
//recursion examples in C
#include <stdio.h>
int factorial(int n);
int findsum(int n);
int findpower(int a, int b);
int factorial(int n)
{
if(n==0)
return 1;
else
return(n*factorial(n-1));
}
int findsum(int n)
{
if(n==0)
return 0;
else
return n+findsum(n-1);
}
int findpower(int a, int b)
{
if(b==0)
return 1;
else
return a*findpower(a, --b);
}
int main()
{
int n, f, sum, a, b, power;
printf("Enter a number to find its factorial: ");
scanf("%d", &n);
f=factorial(n);
printf("Factorial of %d is %d!", n, f);
printf("\n\nEnter a number: ");
scanf("%d", &n);
sum=findsum(n);
printf("\nSum of first %d integers is %d\n", n, sum);
printf("\n Enter two numbers: ");
scanf("%d%d",&a, &b);
power=findpower(a, b);
printf("\n%d raise to the power %d is %d", a, b, power);
return 0;
}
Output
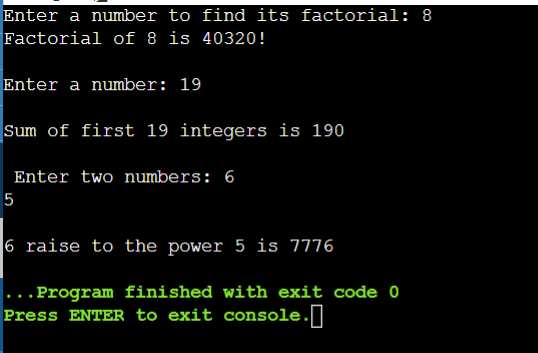
Further Reading
50+ C Programming Interview Questions and Answers
C Program to Find Factorial of a Number
- AI
- Android
- Angular
- ASP.NET
- Augmented Reality
- AWS
- Bioinformatics
- Biometrics
- Blockchain
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Cyber Security
- Data Science
- Data Structures and Algorithms
- Data Visualization
- Datafication
- Deep Learning
- DevOps
- Digital Forensic
- Digital Trust
- Digital Twins
- Django
- Docker
- Dot Net Framework
- Drones
- Elasticsearch
- ES6
- Extended Reality
- Flutter and Dart
- Full Stack Development
- Git
- Go
- HTML
- Image Processing
- IoT
- IT
- Java
- JavaScript
- Kotlin
- Latex
- Machine Learning
- MEAN Stack
- MERN Stack
- Microservices
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Quantum Computing
- React
- Robotics
- Rust
- Scratch 3.0
- Shell Script
- Smart City
- Software
- Solidity
- SQL
- SQLite
- Tecgnology
- Tkinter
- TypeScript
- VB.NET
- Virtual Reality
- Web Designing
- WebAssembly
- XML