The following article describes How to Implement Session Management in JSP.
Session management in JSP (JavaServer Pages) involves several steps. Here is a basic overview of the steps involved in implementing session management.
Create a session object: You can create a session object using the HttpSession interface. This interface provides methods to get and set session attributes, as well as to invalidate the session when it is no longer needed.
HttpSession session = request.getSession();
Set session attributes: You can set session attributes using the setAttribute() method of the HttpSession interface. This method takes a name-value pair as its parameters, where the name is a string that identifies the attribute and the value is the attribute itself.
session.setAttribute(“username”, “john”);
Get session attributes: You can retrieve session attributes using the getAttribute() method of the HttpSession interface. This method accepts the attribute name as its parameter and returns the attribute value.
String username = (String) session.getAttribute(“username”);
Invalidate the session: You can invalidate the session using the invalidate() method of the HttpSession interface. This method removes all session attributes and marks the session as invalid so that it can no longer be used.
session.invalidate();
Here is an example of how to implement session management in a JSP.
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<%
// Get the session object
HttpSession session = request.getSession();
boolean loggedIn = (session.getAttribute("username") != null);
if (loggedIn) {
String username = (String) session.getAttribute("username");
%>
<h2>Welcome <%= username %>!</h2>
<p><a href="logout.jsp">Log out</a></p>
<%
} else {
// If the user is not logged in, display a login form
%>
<h2>Login</h2>
<form action="login.jsp" method="post">
<label>Username:</label>
<input type="text" name="username"><br>
<label>Password:</label>
<input type="password" name="password"><br>
<input type="submit" value="Log in">
</form>
<%
}
%>
In this example, the JSP page checks if the username attribute is set in the session. In this case, it displays a welcome message is displayed with a link to log out. Otherwise, a login form is displayed. When the user submits the form, the login.jsp page can retrieve the submitted username and password, validate them, and set the username attribute in the session if they are valid.
Further Reading
Spring Framework Practice Problems and Their Solutions
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
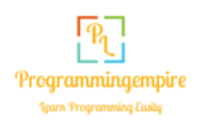