The following article describes How to Display Two-Dimensional Associative Array in PHP.
In order to display a two-dimensional associative array in PHP, you can use a loop to iterate through each element of the array and print out its key-value pairs. The following code example demonstrates a 2D associative array.
$array = array(
array("name" => "John", "age" => 30),
array("name" => "Mary", "age" => 25),
array("name" => "Bob", "age" => 35)
);
foreach ($array as $item) {
foreach ($item as $key => $value) {
echo "$key: $value<br>";
}
echo "<br>";
}
In this example, we have a two-dimensional array with three elements, each containing a “name” and “age” key-value pair. So, the outer loop iterates through each element of the array. Whereas, the inner loop iterates through each key-value pair within that element. Also, the echo statement inside the inner loop prints out each key-value pair separated by a colon, and the “<br>” tag creates a line break after each pair. Moreover, the outer loop also adds an extra line break between each element for clarity.
When you run this code, you should see the following output.
name: John
age: 30
name: Mary
age: 25
name: Bob
age: 35
So, this output shows each key-value pair in the array, separated by a colon and a space, with each element separated by a blank line.
Further Reading
Examples of Array Functions in PHP
Exploring PHP Arrays: Tips and Tricks
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
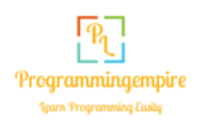