The following article describes How to Display Nested Associative Array in PHP.
In order to display a 2D associative array in PHP, you can use nested loops to iterate over each key-value pair at each level and output them in a readable format. The following example shows the use of two foreach
loops for displaying a nested associative array.
$employees = array(
"John" => array(
"age" => 30,
"department" => "Sales"
),
"Jane" => array(
"age" => 35,
"department" => "Marketing"
)
);
foreach ($employees as $name => $data) {
echo $name . ":<br>";
foreach ($data as $key => $value) {
echo "- " . $key . ": " . $value . "<br>";
}
}
The following output will be shown.
John:
- age: 30
- department: Sales
Jane:
- age: 35
- department: Marketing
As can be seen, in the outer foreach
loop, the $name
variable represents the current key at the first level and the $data
variable represents the nested associative array. So, the loop iterates over each key-value pair at the first level and outputs the name followed by a colon.
Likewise, in the inner foreach
loop, the $key
variable represents the current key at the second level and the $value
variable represents the corresponding value. Also, here the loop iterates over each key-value pair at the second level and outputs the key and value preceded by a hyphen for each employee.
Furthermore, you can use any HTML tags or formatting within the echo
statement to customize the output as desired.
Further Reading
Examples of Array Functions in PHP
Exploring PHP Arrays: Tips and Tricks
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
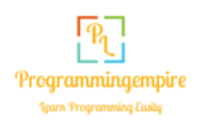