The following article describes How to Display Associative Arrays in PHP.
In order to display an associative array in PHP, you can use a loop to iterate over each key-value pair and output them in a readable format. The following example shows how to use the foreach
loop.
$person = array(
"name" => "John",
"age" => 25,
"city" => "New York"
);
foreach ($person as $key => $value) {
echo $key . ": " . $value . "<br>";
}
This will output following.
name: John
age: 25
city: New York
In the foreach
loop, the $key
variable represents the current key and the $value
variable represents the current value. The loop iterates over each key-value pair in the associative array and outputs them using the echo
statement. You can use any HTML tags or formatting within the echo
statement to customize the output as desired.
Further Reading
Examples of Array Functions in PHP
Exploring PHP Arrays: Tips and Tricks
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
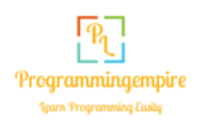