The following example code demonstrates How to Create LinkedList of Numeric Types in Java.
Here is an example Java program that creates a linked list of numeric types.
import java.util.LinkedList;
public class Main {
public static void main(String[] args) {
LinkedList<Number> linkedList = new LinkedList<>();
linkedList.add(10);
linkedList.add(10.5f);
linkedList.add(100.5);
linkedList.add(1000L);
linkedList.add((byte) 50);
for (Number num : linkedList) {
System.out.println(num);
}
}
}
This program creates a linked list of type Number
called linkedList
, adds 5 elements of different numeric types (int
, float
, double
, long
, and byte
) to it, and then displays the elements of the list using a for-each loop. The linked list is created using the LinkedList
class, and elements are added to it using the add
method.
Further Reading
Understanding Enterprise Java Beans
- Android
- Angular
- ASP.NET
- AWS
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Deep Learning
- Django
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- Machine Learning
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- Solidity
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
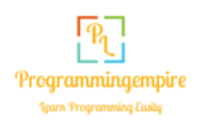