The following code example demonstrates How to Create and Use Abstract Classes in Java.
Basically, an abstract class is created to define some common details that its subclasses can use. For the purpose of creating common attributes and behavior that some specialized classes can use, we create an abstract class. Therefore, abstract classes don’t have complete information to create an object. In other words, an abstract can not be instantiated. It means we can’t create objects of an abstract class. However, we can create concrete subclasses of the abstract class that can be instantiated further.
In Java, we use the abstract keyword to create an abstract class. The following code shows an example of the abstract class Compartment that has an abstract method notice(). Since it is an abstract method, it doesn’t contain a method body. Furthermore, we create four subclasses of the abstract class Compartment – FirstClass, Ladies, General, and Luggage. Evidently, all of these classes are concrete classes. Because these classes inherit from the abstract class Notice, we must define the method notice() in these classes.
Calling the Method of Abstract Class
The following example also demonstrates runtime polymorphism in Java. In the main() method, we create an array of ten elements of the abstract class Compartment. These elements represent the reference variables of the class Compartment. In order to create objects of the concrete subclasses, we generate a random number in the range 1 to 4 in a for loop that iterates ten times. Accordingly, each reference variable in the array of compartment class is assigned an object of a specific subclass. After that, the method notice() is called for each of the elements of the array in another for loop.
Hence, on the basis of the type of object, the corresponding version of the method notice() is called.
abstract class Compartment
{
public abstract String notice();
}
class FirstClass extends Compartment
{
public String notice()
{
return "This is the First Class Compartment!";
}
}
class Ladies extends Compartment
{
public String notice()
{
return "This is the Ladies Compartment!";
}
}
class General extends Compartment
{
public String notice()
{
return "This is the General Compartment!";
}
}
class Luggage extends Compartment
{
public String notice()
{
return "This is the Luggage Compartment!";
}
}
public class TestCompartment
{
public static void main(String[] args)
{
Compartment[] c_arr=new Compartment[10];
for(int i=0;i<10;i++)
{
int a_number=(int)(Math.random()*4);
switch(a_number)
{
case 0: c_arr[i]=new FirstClass(); break;
case 1: c_arr[i]=new Ladies(); break;
case 2: c_arr[i]=new General(); break;
case 3: c_arr[i]=new Luggage(); break;
}
}
for(int i=0;i<10;i++)
{
System.out.println(c_arr[i].notice());
}
}
}
Output
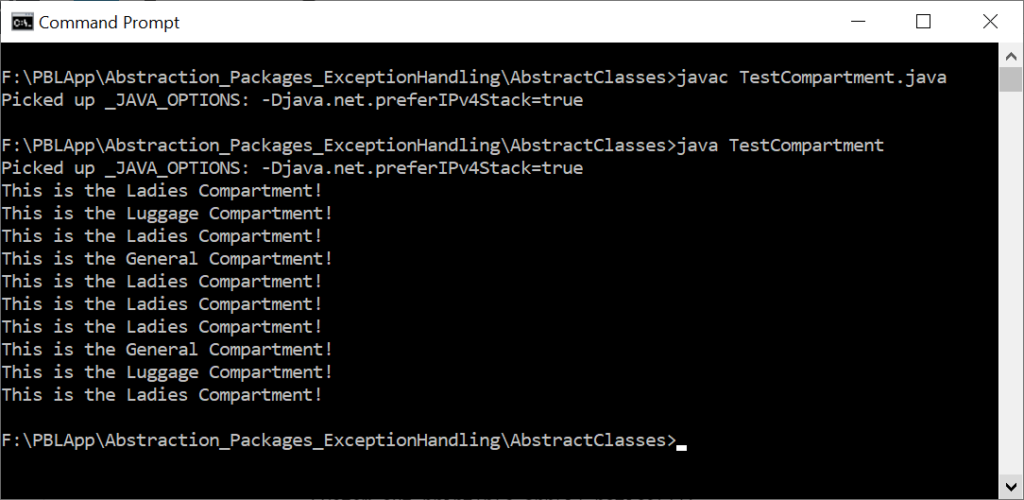
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
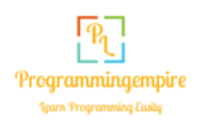