The following example code demonstrates How to Create and Display Cookies Using Servlets.
Here is an example of a dynamic web application using servlets to create and display cookies in Java.
import java.io.*;
import javax.servlet.*;
import javax.servlet.http.*;
public class CookieServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String name = request.getParameter("name");
if (name != null) {
Cookie cookie = new Cookie("name", name);
cookie.setMaxAge(30 * 24 * 60 * 60); // 30 days
response.addCookie(cookie);
response.sendRedirect("/display-cookie");
} else {
RequestDispatcher dispatcher = request.getRequestDispatcher("/create-cookie.jsp");
dispatcher.forward(request, response);
}
}
}
import java.io.*;
import javax.servlet.*;
import javax.servlet.http.*;
public class DisplayCookieServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
Cookie[] cookies = request.getCookies();
String name = null;
if (cookies != null) {
for (Cookie cookie : cookies) {
if (cookie.getName().equals("name")) {
name = cookie.getValue();
break;
}
}
}
request.setAttribute("name", name);
RequestDispatcher dispatcher = request.getRequestDispatcher("/display-cookie.jsp");
dispatcher.forward(request, response);
}
}
Here is an example of the JSP page, create-cookie.jsp
.
<!DOCTYPE html>
<html>
<head>
<title>Create Cookie</title>
</head>
<body>
<h1>Create Cookie</h1>
<form action="/" method="get">
<div>
<label for="name">Name:</label>
<input type="text" id="name" name="name">
</div>
<div>
<button type="submit">Create Cookie</button>
</div>
</form>
</body>
</html>
Here is an example of the JSP page, display-cookie.jsp
.
<!DOCTYPE html>
<html>
<head>
<title>Display Cookie</title>
</head>
<body>
<h1>Display Cookie</h1>
<p>Name: ${name}</p>
</body>
</html>
In this example, the CookieServlet
class creates a cookie and adds it to the response if the name parameter is not null. If the name parameter is null, the request is forwarded to the create-cookie.jsp
page, which allows the user to enter a name. The DisplayCookieServlet
class retrieves the cookies.
Further Reading
Understanding Enterprise Java Beans
- Android
- Angular
- ASP.NET
- AWS
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Deep Learning
- Django
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- Machine Learning
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- Solidity
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
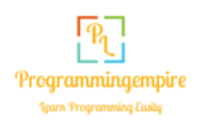