The following article describes How to Access Parameters in JSP.
In a JSP (JavaServer Pages) page, you can access request parameters sent from a client-side form or URL query string using the request.getParameter() method. For example.
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Accessing Parameters in JSP</title>
</head>
<body>
<h1>Accessing Parameters in JSP</h1>
<%-- Get the value of the "name" parameter --%>
<% String name = request.getParameter("name"); %>
<%-- Check if the "name" parameter is present --%>
<% if (name != null && !name.isEmpty()) { %>
<p>Hello, <%= name %>!</p>
<% } else { %>
<p>Please enter your name:</p>
<form action="<%= request.getRequestURI() %>" method="get">
<input type="text" name="name">
<input type="submit" value="Submit">
</form>
<% } %>
</body>
</html>
In this example, the JSP page uses the request.getParameter() method to retrieve the value of the name parameter, which is passed in the query string of the URL. If the name parameter is present and not empty, the JSP page displays a personalized greeting with the value of the name parameter. Otherwise, it displays a form to prompt the user to enter their name. When the form is submitted, the JSP page reloads with the new name parameter in the query string.
Note that the getParameter() method returns a string, so you may need to convert the value to another data type if necessary. Additionally, it’s important to validate user input and handle errors when accessing parameters in a JSP.
Further Reading
Spring Framework Practice Problems and Their Solutions
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
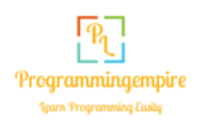