The following article explains how do extension functions and properties work in Kotlin.
In fact, this feature of Kotlin allows you to add new functionality to existing classes without having to modify the original class or create a subclass. Furthermore, we can define them outside of the class. Also, we can call them as if they were methods or properties of the original class.
Examples Demonstrating How do extension functions and properties work in Kotlin
The following example demonstrates the extension function in Kotlin.
Extension Functions
fun String.reverse(): String {
return this.reversed()
}
As can be seen, this function extends the String
class by adding a new method called reverse()
that returns a reversed version of the string. In order to use this function, you can simply call it on a string object. For example.
val str = "Hello, world!"
val reversed = str.reverse() // reversed = "!dlrow ,olleH"
So, the reverse()
function can be called on any string object, even if it was not defined in the original String
class.
Extension Properties
In addition to extension functions, Kotlin also supports extension properties, which allow you to add new properties to existing classes. The following example demonstrates an extension property in Kotlin.
val String.firstChar: Char
get() = this[0]
This extension property extends the String
class by adding a new property called firstChar
that returns the first character of the string. To use this property, you can simply access it as if it were a property of the string:
val str = "Hello, world!"
val firstChar = str.firstChar // firstChar = 'H'
To summarize, it is a very important and powerful feature in Kotlin that can help you write more expressive and concise code. Moreover, they are particularly useful when you need to add new functionality to a class that you don’t control, such as classes from external libraries.
Further Reading
- Android
- Angular
- ASP.NET
- AWS
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Data Science
- Data Structures and Algorithms
- Deep Learning
- Django
- Dot Net Framework
- Drones
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- Machine Learning
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- Smart City
- Software
- Solidity
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
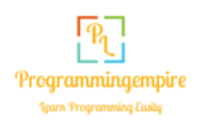