The following program demonstrates how to Find Whether Each Digit of a Number has the Same Frequency.
To begin with, first, we convert the given number into a string. After that, we create an array of characters using that string.
In order to find whether each digit of the number has the same frequency, first, we create an ArrayList object. The ArrayList maintains a list of CharFrequencies objects. The CharFrequencies class has two data members – the character and its frequency. After that, using an iterator, we can find whether all the frequency values are the same or not. Accordingly, a true or false value is returned.
import java.util.*;
public class SameFrequencies
{
public static void main(String[] args)
{
System.out.println(findSameFrequencies(66786));
System.out.println(findSameFrequencies(777888));
System.out.println(findSameFrequencies(735));
System.out.println(findSameFrequencies(122112));
}
public static boolean findSameFrequencies(int n)
{
System.out.println("Number = "+n);
String str=Integer.toString(n);
char[] ch=new char[str.length()];
for(int i=0;i<str.length();i++)
{
ch[i]=str.charAt(i);
}
ArrayList<CharFrequencies> list=new ArrayList<CharFrequencies>();
try{
list.add(new CharFrequencies(ch[0], 1));
for(int i=1;i<ch.length;i++)
{
boolean found=false;
char c1=ch[i];
Iterator<CharFrequencies> it =list.iterator();
while (it.hasNext()) {
CharFrequencies cob = it.next();
if (cob.getC() == c1) {
cob.f++;
found=true;
}
}
if(!found)
{
list.add(new CharFrequencies(ch[i], 1));
}
}
}catch(Exception e){ System.out.println(e.getMessage());
}
boolean sameFreq=true;
CharFrequencies cob1=list.get(0);
int my_f=cob1.getF();
Iterator<CharFrequencies> it2 =list.iterator();
while (it2.hasNext()) {
CharFrequencies cob2 = it2.next();
if(cob2.getF() != my_f)
{
sameFreq=false;
break;
}
}
return sameFreq;
}
}
class CharFrequencies
{
public char c;
public int f;
public CharFrequencies(char c, int f)
{
this.c=c;
this.f=f;
}
public char getC()
{
return this.c;
}
public int getF()
{
return this.f;
}
}
Output

Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
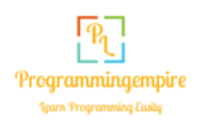