The following program demonstrates how to Find Whether a Number is a Palindrome or Not in Java.
In order to find whether a number is a palindrome or not just need to find its reverse. After that, we can compare both the number and its reverse. If both are the same, then the number is a palindrome.
The following link contains the program to find the reverse of a number.
https://www.programmingempire.com/find-the-reverse-of-a-number-in-java/
import java.util.Scanner;
import java.lang.Math;
public class Exercise17
{
public static void main(String[] args) {
int num, n, i, sum, len, rem;
System.out.println("Enter a Number: ");
num=(new Scanner(System.in)).nextInt();
len=0;
n=num;
while(n>0)
{
n=n/10;
len++;
}
sum=0;
i=len-1;
n=num;
while(n>0)
{
rem=n%10;
sum=sum+rem*(int)(Math.pow(10, i));
n=n/10;
i=i-1;
}
if(sum==num)
{
System.out.println(num+" is a palindrome");
}
else
{
System.out.println(num+" is not a palindrome");
}
}
}
Output
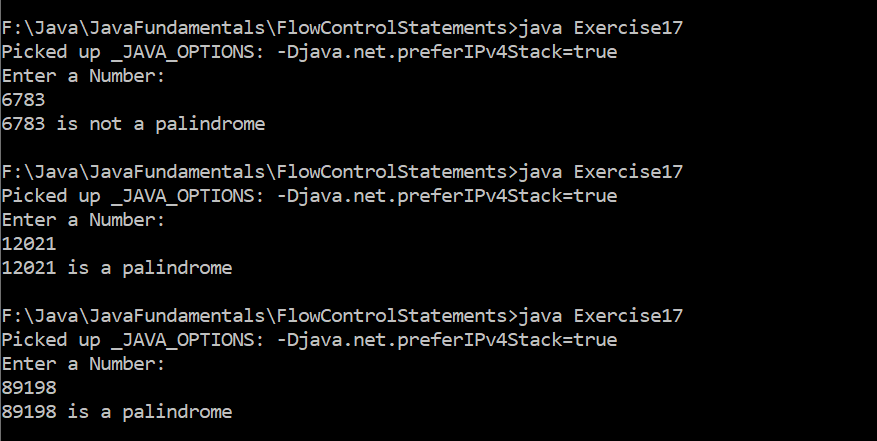
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
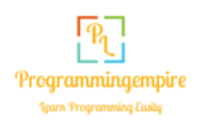