The following program shows how to Find the Type of Character in Java.
In this program, a character variable c1 is declatered and initialized with a value. Further, we assign it to an integer variable n1. Now n1 has its ASCII value. The nested if statement checks whether its value lies in the range 48 to 57, which indicates a digit between 0 to 9. After that, the else part checks whether the given character lies in the range of 65 to 89 or 97 to 122. Because it represents alphabet of uppercase and lowercase respectively. Otherwise, it is a special character. Accordingly, the output displays ‘Digit’, ‘Alphabet’, or ‘Special Character’.
public class Exercise5
{
public static void main(String[] args) {
char c1;
int n1;
c1='T';
n1=c1;
if(n1>=48 && n1<=57)
System.out.println("Digit");
else
if((n1>=65 && n1<=89) ||(n1>=97 && n1<=122))
System.out.println("Alphabet");
else
System.out.println("Special Character");
}
}
Output

Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
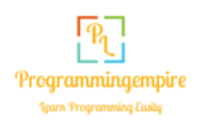