The following code shows how to Find the Sum of Two numbers Using Command Line Arguments in Java.
Since the command line arguments in java are accepted as strings in a string array, we need to convert them into integers. For this purpose, we can use the parseInt() method of the wrapper class Integer before computing the sum.
public class Exercise3
{
public static void main(String[] args) {
int a, b;
if(args.length!=2)
{
System.out.println("Wrong Number of Command Line Arguments!\nExiting...");
System.exit(0);
}
a=Integer.parseInt(args[0]);
b=Integer.parseInt(args[1]);
System.out.println("Sum of "+a+" and "+b+" is "+(a+b));
}
}
Output

Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
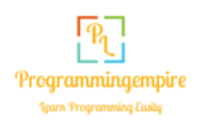