The following article demonstrates how to Find the Sum of Two Matrices in PHP.
In order to add two matrices in PHP, you need to perform element-wise addition of the two matrices. The following code example demonstrates its implementation.
// Define two matrices
$matrix1 = array(
array(1, 2, 3),
array(4, 5, 6),
array(7, 8, 9)
);
$matrix2 = array(
array(9, 8, 7),
array(6, 5, 4),
array(3, 2, 1)
);
// Get the dimensions of the matrices
$rows = count($matrix1);
$cols = count($matrix1[0]);
// Initialize a result matrix
$result = array();
for ($i = 0; $i < $rows; $i++) {
for ($j = 0; $j < $cols; $j++) {
$result[$i][$j] = $matrix1[$i][$j] + $matrix2[$i][$j];
}
}
// Print the result matrix
echo "<table>";
foreach ($result as $row) {
echo "<tr>";
foreach ($row as $value) {
echo "<td>" . $value . "</td>";
}
echo "</tr>";
}
echo "</table>";
As can be seen in this example, we define two matrices $matrix1
and $matrix2
with the same dimensions. Then, we use the count() function to fetch the total number of rows and columns respectively. After that, we initialize an empty array $result
to store the result matrix.
Then, we iterate over each element of the matrices using two nested loops and add the corresponding elements of $matrix1
and $matrix2
to get the corresponding element of the result matrix $result
.
Finally, we print the result matrix in tabular format using nested loops.
The following output will be generated.
10 10 10
10 10 10
10 10 10
Also, note that the values in the result matrix are the sum of the corresponding elements of the input matrices.
Further Reading
Examples of Array Functions in PHP
Exploring PHP Arrays: Tips and Tricks
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
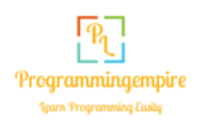