The following program demonstrates how to Find the Reverse of a Two Dimensional Array in Java.
Basically, here we create a 2 X 2 array by taking four integers as command line arguments. If the user doesn’t provide exactly four integers, the program shows an error message and exits. Otherwise, it creates a 2D array y using these numbers. Further, a new 2 X 2 array is created and the nested for loop inserts the elements of the original array into the new array in reverse order.
public class Exercise13
{
public static void main(String[] args) {
if(args.length!=4)
{
System.out.println("Please enter 4 integer numbers");
System.exit(0);
}
int[][] arr=new int[2][2];
int[][] arr1=new int[2][2];
int c=0;
for(int i=0;i<2;i++)
{
for(int j=0;j<2;j++)
{
arr[i][j]=Integer.parseInt(args[c]);
c++;
}
}
System.out.println("Original Array...");
for(int i=0;i<2;i++)
{
for(int j=0;j<2;j++)
{
System.out.print(arr[i][j]+" ");
}
System.out.println();
}
for(int i=0;i<2;i++)
{
for(int j=0;j<2;j++)
{
arr1[i][j]=arr[1-i][1-j];
}
System.out.println();
}
arr=arr1;
System.out.println("Reversed Array...");
for(int i=0;i<2;i++)
{
for(int j=0;j<2;j++)
{
System.out.print(arr[i][j]+" ");
}
System.out.println();
}
}
}
Output

Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
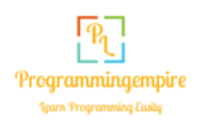