The following program demonstrates how to Find Possible Strings to Complete a Word in Java.
Suppose, you have a word, which has a single character missing in it. For example, suppose the word is c_T. Now you need to find, the possible words in a string that would complete the above word by replacing the ‘_’ character with another single character. Another input to this function is a string that contains certain words separated by the ‘:’. You have to find which of these words can form the given word by replacing ‘_’ with a single character.
For example, if the string is “CIT:COTT:cot:Cat”, the possible words for c_T are CIT, cot, and Cat. The output of this program should be a single string containing all the possible words separated by a ‘:’. In other words, the output in the above example is CIT:cot:Cat.
public class Possible
{
public static void main(String[] args)
{
System.out.println(findPossible("Fi_er", "Fever:filer:Filter:Fixer:fiber:fibre:tailor:offer"));
System.out.println(findPossible("c_T", "Cut:Bit:ciit:caT:cot:cutt:puTT:CIT"));
}
public static String findPossible(String input1, String input2)
{
input1=input1.toLowerCase();
input2=input2.toLowerCase();
String[] sarr=input2.split(":");
String str=input1, out_str="";
boolean found=false;
char[] input_ch=new char[str.length()];
System.out.println(str);
for(int i=0;i<str.length();i++)
{
input_ch[i]=str.charAt(i);
}
for(int i=0;i<sarr.length;i++)
{
String next=sarr[i];
//System.out.println(next);
char[] next_ch=new char[next.length()];
for(int j=0;j<next.length();j++)
{
next_ch[j]=next.charAt(j);
}
int k=0, m=0;
while(input_ch[k++]==next_ch[m++]);
if(input_ch[k]=='_')
{
m++;
}
else
{
found=false;
}
while(k<input_ch.length)
{
if(input_ch[k]!=next_ch[m])
{
found=false;
break;
}
k++;
m++;
}
if((k==input_ch.length) && (m==next_ch.length))
found=true;
if(found)
out_str+=next.toUpperCase()+":";
//System.out.println(out_str);
found=false;
}
if(out_str.length()==0) return "ERROR-009";
return out_str.substring(0, out_str.length()-1);
}
}
Output
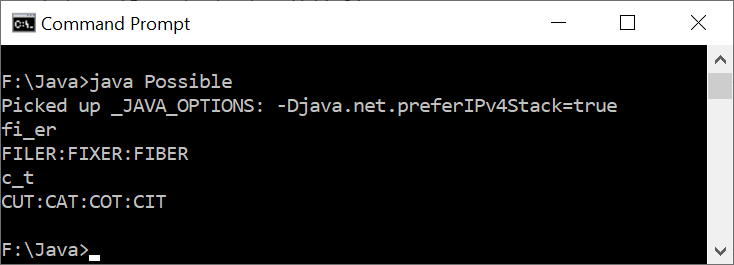
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
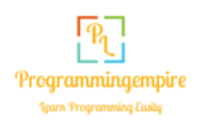