The following code shows how to Find Factorial of a Number using Recursion in C Language.
// Recursive Function to Find Factorial of a Number
#include <stdio.h>
int recursive_factorial(int n)
{
if(n==0)
return 1;
else
return n*recursive_factorial(n-1);
}
int main()
{
int mynumber, result;
printf("Enter a Number: ");
scanf("%d", &mynumber);
result=recursive_factorial(mynumber);
printf("Factorial of %d is %d", mynumber, result);
return 0;
}
Output
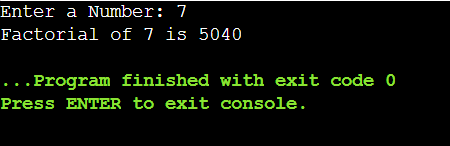
Further Reading
50+ C Programming Interview Questions and Answers
C Program to Find Factorial of a Number
- AI
- Android
- Angular
- ASP.NET
- Augmented Reality
- AWS
- Bioinformatics
- Biometrics
- Blockchain
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Cyber Security
- Data Science
- Data Structures and Algorithms
- Data Visualization
- Datafication
- Deep Learning
- DevOps
- Digital Forensic
- Digital Trust
- Digital Twins
- Django
- Docker
- Dot Net Framework
- Drones
- Elasticsearch
- ES6
- Extended Reality
- Flutter and Dart
- Full Stack Development
- Git
- Go
- HTML
- Image Processing
- IoT
- IT
- Java
- JavaScript
- Kotlin
- Latex
- Machine Learning
- MEAN Stack
- MERN Stack
- Microservices
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Quantum Computing
- React
- Robotics
- Rust
- Scratch 3.0
- Shell Script
- Smart City
- Software
- Solidity
- SQL
- SQLite
- Tecgnology
- Tkinter
- TypeScript
- VB.NET
- Virtual Reality
- Web Designing
- WebAssembly
- XML