Here we discuss several Examples of Using Static Members in Java.
Basically, java provides a static keyword that we can use in four different ways. Accordingly, we have static variables, static methods, static class, and static block.
The following code presents an example of Static Members in Java. It includes the usage of static variables.
In fact, static variables represent class variables. Hence, these variables are common for all objects of the class. Furthermore, they are allocated a single location in memory. Therefore, when any object updates a static variable, all other objects can access the updated value. As can be seen in the following example, the variable mycounter is a static variable. When we create an object of the class, its constructor executes and increments mycounter. This incremented value of mycounter is available to all objects of the class.
class MyStaticMembers
{
public static int mycounter=0;
public MyStaticMembers()
{
mycounter++;
}
}
public class Main
{
public static void main(String[] args) {
MyStaticMembers ob1, ob2, ob3, ob4;
ob1=new MyStaticMembers();
System.out.println("Counter = "+ob1.mycounter);
ob2=new MyStaticMembers();
System.out.println("Counter = "+ob2.mycounter);
ob3=new MyStaticMembers();
System.out.println("Counter = "+ob3.mycounter);
ob4=new MyStaticMembers();
System.out.println("Counter = "+ob4.mycounter);
}
}
Output

Using Static Members in Java – Static Methods
Like static variables, we can also create static methods. Static methods belong to the class rather than individual objects. Further, it is possible o call a static method without creating an object of the class. Because, we can call the static method using the class name and the dot operator.
The following program shows the use of a static method. As can be seen, we declare a static method with the keyword static. Also, we call it by using the class name.
class MyStaticMembers
{
static int mycounter=0;
public MyStaticMembers()
{
mycounter++;
}
public static void StaticMethod()
{
System.out.println("Counter = "+mycounter);
}
}
public class Main
{
public static void main(String[] args) {
MyStaticMembers ob1, ob2, ob3, ob4;
ob1=new MyStaticMembers();
ob2=new MyStaticMembers();
ob3=new MyStaticMembers();
ob4=new MyStaticMembers();
MyStaticMembers.StaticMethod();
}
}
Output
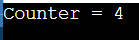
Similarly, Java also also provides us a way of initialising static variables. So, we have static blocks in Java to assign the values to static variables. An example of using static block is given here.
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
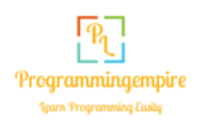