The following programs demonstrate some Examples of Creating and Using Tuples in Python.
In fact, a tuple is one of the collections in python. A tuple may contain elements of different data types. The following example shows how to create a tuple. Also, the type of tuple is displayed here.
Example 1
t1 = ()
print(t1)
t2 = (10)
print(type(t1))
print(t2)
t3 = (10,1.2,'d',"Ram")
print(type(t3))
print(t3)
Output
() <class 'tuple'> 10 <class 'tuple'> (10, 1.2, 'd', 'Ram')
Example 2
Similarly, the following example also shows creation of a tuple.
t4 = 10,1.2,'d',"Ram"
print(type(t4))
print(t4)
Output
<class 'tuple'> (10, 1.2, 'd', 'Ram')
Example 3
Another example of creating a tuple.
a,b= 10,20
print(a,b)
Output
10 20
Example 4
The following example shows passing a tuple to a function.
def max_min(t):
x = max(t)
y = min(t)
return (x,y)
t = (2,4,1,10,56,5)
(Max,Min) = max_min(t)
print(Max,Min)
Output
56 1
Example 5
Similarly, a tuple may contain other tuples. The following example demonstrates it.
Toppers = (("Ram", "BCA", 92),("Mohan", "BBA", 93),("Mukesh", "MCA", 91))
for i in Toppers:
print(i)
Output
('Ram', 'BCA', 92) ('Mohan', 'BBA', 93) ('Mukesh', 'MCA', 91)
Example 6
The folowing example shows creating a tuple and printing its individual elements.
Toppers = (("Ram", "BCA", 92),("Mohan", "BBA", 93),("Mukesh", "MCA", 91))
print("%s is a student of %s got aggreagte marks %d" %(Toppers[0][0],Toppers[0][1],Toppers[0][2]))
Output
Ram is a student of BCA got aggreagte marks 92
Example 7
Likewise, the following example prints a tuple in a loop.
Toppers = (("Ram", "BCA", 92),("Mohan", "BBA", 93),("Mukesh", "MCA", 91))
for i,j,k in Toppers:
print(i,j,k)
Output
Ram BCA 92 Mohan BBA 93 Mukesh MCA 91
Example 8
However, the following tuple contains other tuples as well as a string as its elements.
Toppers = (("Ram", "BCA",[92,87,96,90]),("Mohan", "BBA", [91,93,98,89]),("Mukesh", "MCA", [90,95,93,89]))
for i in Toppers:
print(i)
print("Name=",Toppers[1][0])
print("Marks=",Toppers[1][2])
Output
('Ram', 'BCA', [92, 87, 96, 90]) ('Mohan', 'BBA', [91, 93, 98, 89]) ('Mukesh', 'MCA', [90, 95, 93, 89]) Name= Mohan Marks= [91, 93, 98, 89]
Example 9
Furthermore, we can apply a user-defined method to each element of the tuple. However, it produces a list. The following example shows it.
def double(T):
return [i*2 for i in T]
T = (1,2,3,4,5)
print("Original Tuple:",T)
print("Double values:",double(T))
Output
Original Tuple: (1, 2, 3, 4, 5) Double values: [2, 4, 6, 8, 10]
Examples of Creating Tuples in Python – Tuple Functions
Basically, tuples in python offer several built-in functions. These functions are len(),min(), max(), sum(), any(), all(), index(), cmp(), reversed(), sorted(), and so on. The folowing examples demonstrate the use of these functions.
Example 10
Infact, zip() function takes two arguments. Furthermore, both of them are iterables. So, te zip() function creates the pairs of each of the correspinding elements in these two iterables. Also, we use the list() function. As a result, the pairs of elements are included in a list. Hence, we get this list as output.
t1 =(1,2,3)
L1 = ['a','b','c']
print(list(zip(t1,L1)))
Output
[(1, 'a'), (2, 'b'), (3, 'c')]
Example 11
The following code shows the use of other functions. While, the index() function returns the index of an element. Similarly, min(), amd max() return the minimum and maximum from the tuple respectively. Whereas, the sum() function returns the sum of all elements in the tuple.
t1= (1,2,3,4,5,6)
print(t1.index(6))
print(min(t1))
print(max(t1))
print(sum(t1))
Output
5 1 6 25
Example 12
following code shows how the count() function returns the count of a specific element in the list.
t1= (1,2,3,4,5,6,1,2,1)
print(t1.count(10))
print(t1.count(1))
print(t1.count(2))
print(t1.count(5))
Output
0 3 2 1
Further Reading
Examples of OpenCV Library in Python
A Brief Introduction of Pandas Library in Python
A Brief Tutorial on NumPy in Python
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
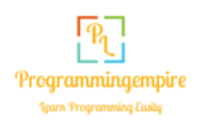