The following article shows some examples of associative arrays in PHP.
In order to create arrays with key/value pairs, we use associative arrays. When the index or subscripts of an array need not be an index, we may use associative arrays. Also, the keys and values represent a relationship in an associative array.
- Example with named keys. The following example shows a simple array representing a person.
$person = array(
"name" => "John",
"age" => 25,
"city" => "New York"
);
- Example with numeric keys. Of course, we can use numeric keys in an associative array, as shown in the following example.
$fruits = array(
0 => "Apple",
1 => "Banana",
2 => "Orange"
);
- Example with mixed keys. The following example demonstrates that the keys need not have the same data type.
$student = array(
"name" => "Mary",
"age" => 21,
2 => "Junior"
);
- Example with nested arrays. When the value itself represents an array, we can use nested arrays. The following example shows that the name of employee is used as the key. While, the value is comprised of age and department.
$employees = array(
"John" => array(
"age" => 30,
"department" => "Sales"
),
"Jane" => array(
"age" => 35,
"department" => "Marketing"
)
);
- Example with dynamic keys. The following example shows the use of dynamic keys.
$colors = array();
$colors["red"] = "#FF0000";
$colors["green"] = "#00FF00";
$colors["blue"] = "#0000FF";
In all these examples, the keys are used to access the corresponding values within the associative array.
Further Reading
Examples of Array Functions in PHP
Exploring PHP Arrays: Tips and Tricks
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
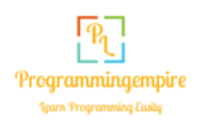