The following program demonstrates an Example of Using TreeSet with User-Defined Objects.
Since here we are creating our TreeSet object with the user-defined objects, we must implement the Comparable interface in the class that will serve as the source to create user-defined objects. In this example, we have a class Emp that contains certain attributes along with the getter and setter methods. The Emp class implements the Comparable interface and defines the compareTo() method.
import java.util.Iterator;
import java.util.TreeSet;
public class TreesetEmpDemo {
public static void main(String[] args) {
// TODO Auto-generated method stub
TreeSet<Emp> ts=new TreeSet<Emp>();
ts.add(new Emp(11, "A", 12000));
ts.add(new Emp(12, "B", 17700));
ts.add(new Emp(13, "C", 18700));
ts.add(new Emp(14, "D", 14500));
ts.add(new Emp(15, "E", 11400));
ts.add(new Emp(16, "F", 12000));
ts.add(new Emp(17, "G", 16700));
System.out.println("Elements in TreeSet: ");
Iterator it=ts.iterator();
while(it.hasNext())
{
System.out.println(it.next().toString());
}
System.out.println("First Element of the TreeSet: "+ts.first());
System.out.println("Last Element of the TreeSet: "+ts.last());
}
}
class Emp implements Comparable<Emp>
{
int emp_id;
String ename;
int salary;
public int compareTo(Emp e2)
{
if (salary==e2.getSalary())
return 0;
else
if(salary>e2.getSalary())
return 1;
else
return -1;
}
public Emp() {
super();
}
public Emp(int emp_id, String ename, int salary) {
super();
this.emp_id = emp_id;
this.ename = ename;
this.salary = salary;
}
public int getEmp_id() {
return emp_id;
}
public void setEmp_id(int emp_id) {
this.emp_id = emp_id;
}
public String getEname() {
return ename;
}
public void setEname(String ename) {
this.ename = ename;
}
public int getSalary() {
return salary;
}
public void setSalary(int salary) {
this.salary = salary;
}
@Override
public String toString() {
return "Employee Details: [emp_id=" + emp_id + ", ename=" + ename + ", salary=" + salary + "]";
}
}
Output
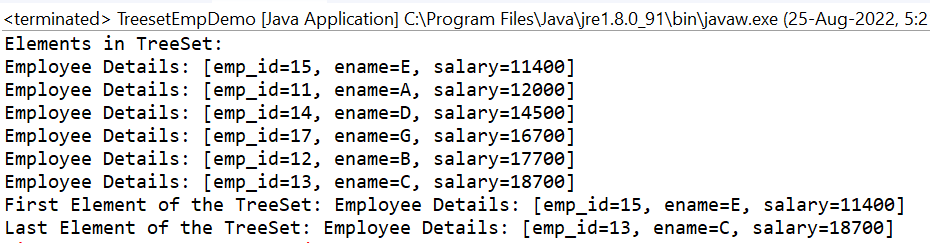
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
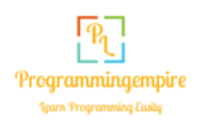