The following code shows an Example of Switch Case Statement in Java.
Here, we use the switch case statement to compute the grade of a student given his marks. In order to accept input from the user, we use the nextInt() method of the Scanner class. Hence, the package java.util need to be imported. Since the valid marks should be in the range of 0 to 100, any value of marks outside this range should result in an error message. Further, we divide the marks by 10 so we get an integer value from 0 to 10.
For the purpose of computing grade, we use the following set of conditions:
80>=marks<=100 Grade: A+
70>=marks<80 Grade: A
60>=marks<70 Grade: B+
50>=marks<60 Grade: B
40>=marks<50 Grade: C
0>=marks<40 Grade: F
The switch case statement evaluates the value of marks and matches it with case statements. When a case label matches, it updates the value of the variable grade and breaks from the switch statement. In case, no case label matches, it executes the default statement.
import java.util.*;
class Grade{
public static void main(String[] args) {
System.out.println("Enter Marks (Out of 100) of the Student: ");
Scanner s=new Scanner(System.in);
int marks=s.nextInt();
marks=marks/10;
String grade="";
switch(marks)
{
case 10:
case 9:
case 8:
grade="A+";
break;
case 7:
grade="A";
break;
case 6:
grade="B+";
break;
case 5:
grade="B";
break;
case 4:
grade="C";
break;
case 3:
case 2:
case 1:
case 0:
grade="F";
break;
default:
System.out.println("Wrong marks entered!");
System.exit(0);
}
System.out.println("Grade is "+grade);
}
}
Output

Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
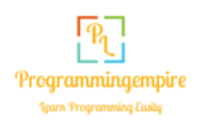