The following program shows a simple Example of Nested If Statement in Java.
In this program, the use of a nested if statement has been demonstrated. The rate of interest on a Fixed Deposit is to be calculated. As can be seen in the code, it requires the user to specify gender and age as command line arguments. In case, the gender is ‘Female’, the age has been checked next. When the age falls in the range 1 to 48, the interest rate is 8.6%. When the age is in the range 49 to 100, the rate is 9.6%. Similarly, when gender is ‘Male’ and age lies in the range 1 to 48, the interest is 7.4%. For the range 49 to 100, the interest rate is 8.7%. The output shows the interest rate in percentage.
public class Exercise6
{
public static void main(String[] args) {
if(args.length!=2)
{
System.out.println("Wrong Number of Command Line Arguments!\nExiting...");
System.exit(0);
}
double interest=0;
String gender=args[0];
int age=Integer.parseInt(args[1]);
if(gender.equals("Female"))
{
if((age>=1) && (age<=48))
{
interest=8.6;
}
else if(age<=100)
interest=9.6;
}
else
{
if(gender.equals("Male"))
{
if((age>=1) && (age<=48))
{
interest=7.4;
}
else if(age<=100)
interest=8.7;
}
else
{
System.out.println("Wrong argument values!");
System.exit(0);
}
}
System.out.println("Interest = "+interest+"%");
}
}
Output
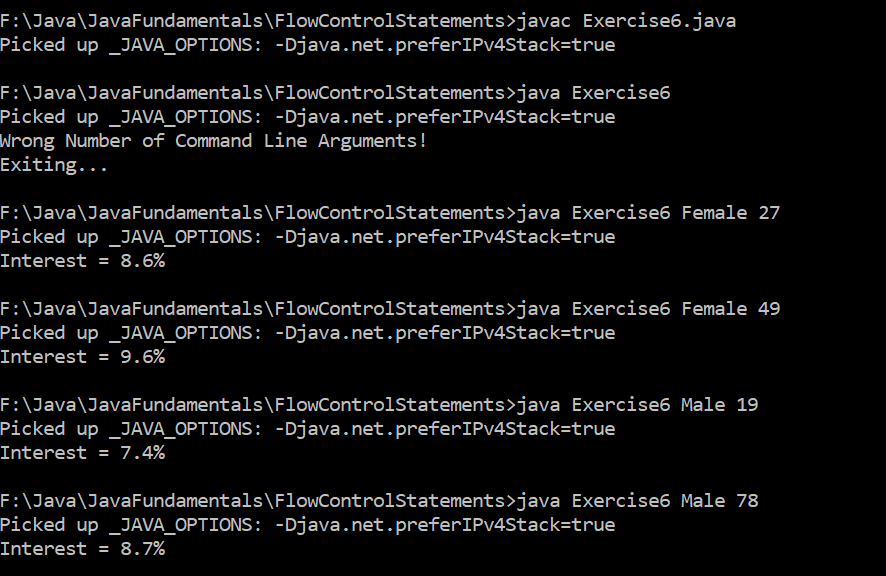
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
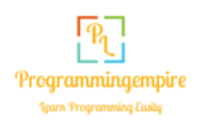