The following code shows an Example of Creating Cookies in PHP.
Cookies offer a convenient way to store a small amount of client information. The following example works as follows. At first, the user enters some values in the text fields in the HTML form. When the user clicks on the Submit button, the if block in the PHP script executes. It calls the setcookie() method to create a cookie with name My_Choices.
Further note that you may need to refresh the web page so that the cookie gets created.
<?php
if(isset($_POST['submit']))
{
$sport=$_POST['t1'];
$book=$_POST['t2'];
$place=$_POST['t3'];
$expiry=time()+12*24*60*60;
$choices=(object)array("Sport"=>$sport, "Book"=>$book, "Place"=>$place);
$cookie_name="My_Choices";
$cookie_info=(object)array("values"=>$choices, "expiry_date"=>$expiry);
setcookie($cookie_name, json_encode($cookie_info), $expiry);
}
echo $_COOKIE['My_Choices'];
$mycookies=json_decode($_COOKIE['My_Choices']);
$info=$mycookies->values;
$s=$mycookies->values->Sport;
$b=$mycookies->values->Book;
$p=$mycookies->values->Place;
$expiry_date=date("Y-m-d H:i:s", $mycookies->expiry_date);
echo "<br>Favourite Sport: ",$s,'<br>';
echo "Favourite Book: ",$b,'<br>';
echo "Favourite Place: ",$p,'<br>';
echo "Expiry Date: ",$expiry_date,'<br>';
?>
<html>
<head>
<title>Creating Cookies</title>
</head>
<body>
<div style="margin:50px;">
<h1>Enter Your Choices</h1>
<form name="myform" action="<?php echo $_SERVER['PHP_SELF']; ?>" method="POST">
Your Favourite Sport: <input type="text" name="t1"><br/><br/>
Your Favourite Book: <input type="text" name="t2"><br/><br/>
Your favourite Place: <input type="text" name="t3"><br/><br/>
<input type="submit" name="submit" value="Submit"><br/>
</form>
</div>
</body>
</html>
Output
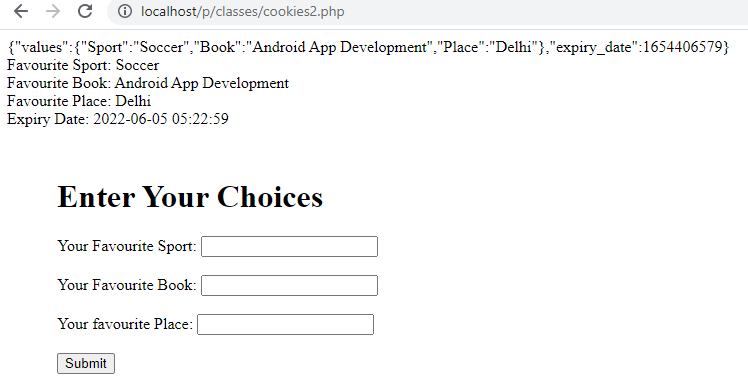
Further Reading
Examples of Array Functions in PHP
- AI
- Android
- Angular
- ASP.NET
- Augmented Reality
- AWS
- Bioinformatics
- Biometrics
- Blockchain
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Cyber Security
- Data Science
- Data Structures and Algorithms
- Data Visualization
- Datafication
- Deep Learning
- DevOps
- Digital Forensic
- Digital Trust
- Digital Twins
- Django
- Docker
- Dot Net Framework
- Drones
- Elasticsearch
- Extended Reality
- Flutter and Dart
- Git
- Go
- HTML
- Image Processing
- IoT
- IT
- Java
- JavaScript
- Kotlin
- Latex
- Machine Learning
- MEAN Stack
- MERN Stack
- Microservices
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Quantum Computing
- React
- Robotics
- Rust
- Scratch 3.0
- Shell Script
- Smart City
- Software
- Solidity
- SQL
- SQLite
- Tecgnology
- Tkinter
- TypeScript
- VB.NET
- Virtual Reality
- Web Designing
- WebAssembly
- XML