The following code shows an Example of Call By Value and Call By Reference in C.
While, call by value in a function refers to calling a function where function parameters are passed by value. In other words, within the function only a copy of the actual parameters is maintained which is separately updated. Hence, the value of actual parameters remain unchanged. For instance, in the function f2(), the value of x and y is copied in separate location and is changed there only. So, the changes are not reflected in actual parameters.
In contrast, when the function is called by reference, the address of function parameters is passed instead of there values. Therefore, all updates within the function are done at actual locations. Hence, when the function returns, updates are reflected in actual parameters. For instance, the function f2() uses call by reference, Therefore, we get the updated values.
// Call By Value and Call By Reference
#include <stdio.h>
void f1(int *a, int *b)
{
*a=*a**b;
*b=*a+*b;
}
void f2(int a, int b)
{
a=a*b;
b=a+b;
}
int main()
{
int x, y;
x=8;
y=12;
printf("Before function call, x=%d, y=%d", x, y);
printf("\nCall By Value....");
f2(x, y);
printf("\nAfter function call, x=%d, y=%d", x, y);
x=8;
y=12;
printf("\nBefore function call, x=%d, y=%d", x, y);
printf("\nCall By Reference....");
f1(&x, &y);
printf("\nAfter function call, x=%d, y=%d", x, y);
return 0;
}
Output
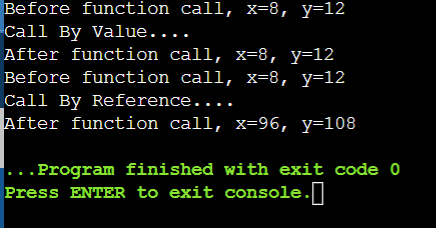
Further Reading
50+ C Programming Interview Questions and Answers
C Program to Find Factorial of a Number
- AI
- Android
- Angular
- ASP.NET
- Augmented Reality
- AWS
- Bioinformatics
- Biometrics
- Blockchain
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Cyber Security
- Data Science
- Data Structures and Algorithms
- Data Visualization
- Datafication
- Deep Learning
- DevOps
- Digital Forensic
- Digital Trust
- Digital Twins
- Django
- Docker
- Dot Net Framework
- Drones
- Elasticsearch
- ES6
- Extended Reality
- Flutter and Dart
- Full Stack Development
- Git
- Go
- HTML
- Image Processing
- IoT
- IT
- Java
- JavaScript
- Kotlin
- Latex
- Machine Learning
- MEAN Stack
- MERN Stack
- Microservices
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Quantum Computing
- React
- Robotics
- Rust
- Scratch 3.0
- Shell Script
- Smart City
- Software
- Solidity
- SQL
- SQLite
- Tecgnology
- Tkinter
- TypeScript
- VB.NET
- Virtual Reality
- Web Designing
- WebAssembly
- XML