The following program demonstrates how to Display the Two Largest and Two Smallest Elements in an Array in Java.
Basically, we find the first largest and first smallest elements as follows. We take two variables maximum, and minimum, and assign the first element of the array to both of them. After that, in a loop, we compare all the subsequent elements with the value of minimum and maximum. In case, the array element is greater than the maximum or less than the minimum, we change the corresponding value of maximum or minimum.
In order to find the second largest element and the second smallest element, we use another for loop. Like before, we initialize the two variables with the first element of the array. However, in the for loop, we compare the second largest with the value of the current element of the array. Also, we need to check whether the array element is smaller than the maximum value. If both of these conditions satisfy, we assign the current array element to the second largest. Similarly, we find the value of second smallest element.
public class Exercise5
{
public static void main(String[] args) {
int[] myarray={3, 5, 1, 12, 9, 24, 6, 8};
int maximum=myarray[0], minimum=myarray[0], second_maximum=myarray[0], second_minimum=myarray[0];
System.out.println("Array Elements...");
for(int i=0;i<myarray.length;i++)
{
System.out.print(myarray[i]+"\t");
if(maximum<myarray[i]) maximum=myarray[i];
if(minimum>myarray[i]) minimum=myarray[i];
}
for(int i=0;i<myarray.length;i++)
{
if((second_maximum<myarray[i]) && (myarray[i]<maximum)) second_maximum=myarray[i];
if((minimum>myarray[i]) && (myarray[i]>minimum)) second_minimum=myarray[i];
}
System.out.println("\nMaximum = "+maximum+"\nMinimum = "+minimum);
System.out.println("\nSecond Maximum = "+second_maximum+"\nSecond Minimum = "+second_minimum);
}
}
Output

Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
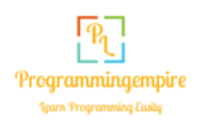