The following program demonstrates how to Display the Sum of Digits of a Number in Java.
In this program, a number is read from the console using an object of the Scanner class. After that, we determine the number of digits in the number by dividing the number by 10 in a while loop. So, the loop runs until the number becomes zero. Also, the variable len is incremented by 1 in each iteration. Hence, at the end of the loop, it contains the number of digits in the given number.
After that, we assign the value of the number in another variable and start another while loop that also iterates until the number becomes zero. In order to compute the sum of digits, we find the remainder by dividing the number by 10. It gives us the last digit that we add to the sum variable. Then we divide the number by 10. When the loop terminates, the sum variable contains the sum of all digits of the number and we display it.
import java.util.Scanner;
import java.lang.Math;
public class Exercise14
{
public static void main(String[] args) {
int num, n, i, sum, len, rem;
System.out.println("Enter a Number: ");
num=(new Scanner(System.in)).nextInt();
len=0;
n=num;
while(n>0)
{
n=n/10;
len++;
}
sum=0;
i=len-1;
n=num;
while(n>0)
{
rem=n%10;
sum+=rem;
n=n/10;
i=i-1;
}
System.out.println(sum);
}
}
Output
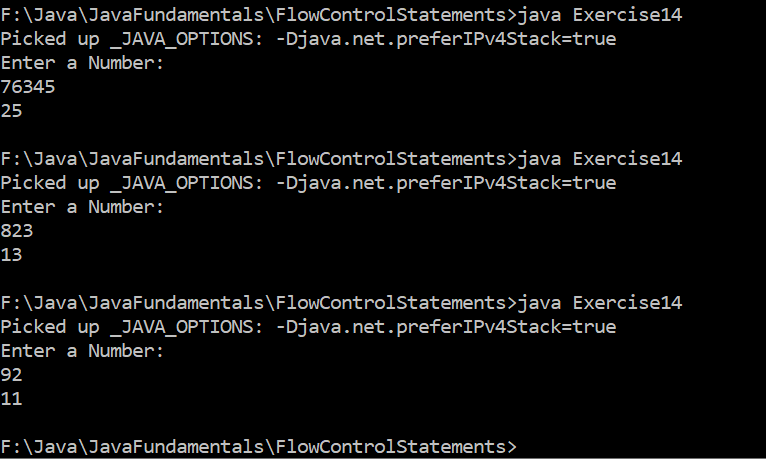
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
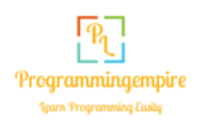