The following program shows how to Display the Sum and Average of All Elements of an Array in Java.
In fact, this program also demonstrates how to initialize an array in Java. After initialization, the for loop computes the sum of elements of the array by reading each element of the array. Further, it computes the average. Also, we need to convert the sum to double data type. Since we need to display the fraction part of the average also.
public class Exercise1
{
public static void main(String[] args) {
int[] myarray={3, 5, 1, 12, 9, 24, 6, 8};
int sum=0;
double average;
System.out.println("Array Elements...");
for(int i=0;i<myarray.length;i++)
{
System.out.print(myarray[i]+"\t");
sum+=myarray[i];
}
average=(double)(sum)/8;
System.out.println();
System.out.println("Sum = "+sum+"\nAverage = "+average);
}
}
Output

Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
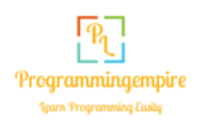