The following program demonstrates how to Display the nth Number in a Sequence in Java.
At first, this program generates a series up to the given number of terms. Then, it returns the nth number in the series. There are four inputs – x1, x2, x3, and x4. Further, x1, x2, and x3 are the three terms in the series. Whereas, x4 represents n. The following conditions should satisfy:
- x1<x2<x3
- Let d be the next element after x3. So, d-x3 should be the same as x2-x1.
- Let e be the next element of d., so, e-d should be equal to x3-x2.
For example, let x=1, x2=3, x3=6, x4=17. So, the 17th number in the series should be returned. Therefore, the series will be
1 3 6 8 11 13 16 18 21 23 26 28 31 33 36 38 41. So, the 17th element is 41. Also, x2-x1=2, and x3-x2=3.
public class Sequences
{
public static void main(String[] args)
{
System.out.println(firstSequence(1, 3, 6, 17));
System.out.println(firstSequence(5, -2, -4, 14));
}
public static int firstSequence(int a, int b, int c, int N)
{
int next=0, t1, t2, t3, diff1, diff2;
t1=a; t2=b; t3=c;
diff1=b-a;
diff2=c-b;
System.out.print(a+" "+b+" "+c+" ");
for(int i=4;i<=N;i++)
{
if(i%2==0)
{
next=t3+diff1;
t1=t2;
t2=t3;
t3=next;
}
else
{
next=t3+diff2;
t1=t2;
t2=t3;
t3=next;
}
System.out.print(next+" ");
}
System.out.println();
return next;
}
}
Output
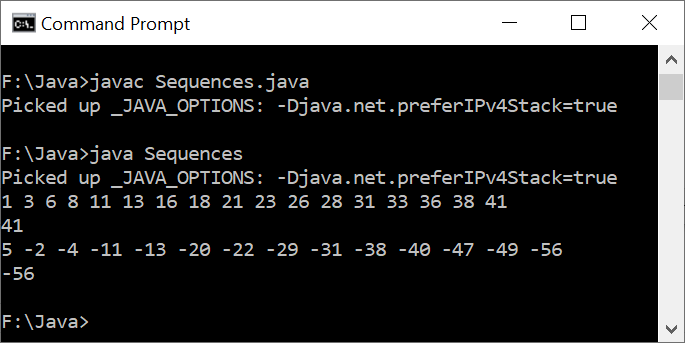
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
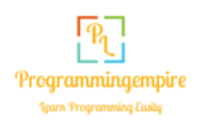