The following example code demonstrates how to Display Data from a Database Table Using Servlets.
Here is an example of a program using servlets to display data from a database table in Java.
import java.io.*;
import java.sql.*;
import javax.servlet.*;
import javax.servlet.http.*;
public class DisplayDataServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
Connection connection = null;
Statement statement = null;
ResultSet resultSet = null;
try {
// Load the JDBC driver
Class.forName("com.mysql.jdbc.Driver");
// Connect to the database
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "root", "password");
// Create a statement
statement = connection.createStatement();
// Execute a query
resultSet = statement.executeQuery("SELECT * FROM employees");
// Store the result set in the request as an attribute
request.setAttribute("resultSet", resultSet);
// Forward the request to the JSP page
RequestDispatcher dispatcher = request.getRequestDispatcher("/display-data.jsp");
dispatcher.forward(request, response);
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
// Close the result set
if (resultSet != null) {
resultSet.close();
}
// Close the statement
if (statement != null) {
statement.close();
}
// Close the connection
if (connection != null) {
connection.close();
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
Similarly, here is an example of the JSP page, display-data.jsp
.
<!DOCTYPE html>
<html>
<head>
<title>Display Data</title>
</head>
<body>
<h1>Display Data</h1>
<table>
<tr>
<th>ID</th>
<th>Name</th>
<th>Email</th>
</tr>
<%
ResultSet resultSet = (ResultSet) request.getAttribute("resultSet");
while (resultSet.next()) {
%>
<tr>
<td><%= resultSet.getInt("id") %></td>
<td><%= resultSet.getString("name") %></td>
<td><%= resultSet.getString("email") %></td>
</tr>
<%
}
%>
</table>
</body>
</html>
In this example, the DisplayDataServlet
class handles GET requests. The servlet loads the JDBC driver, connects to the database, creates a statement, and executes a query to retrieve the data from the employees
table. The result set is stored in the request as an attribute and the request is then forwarded to the display-data.jsp
page,
Further Reading
Understanding Enterprise Java Beans
- Android
- Angular
- ASP.NET
- AWS
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Deep Learning
- Django
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- Machine Learning
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- Solidity
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
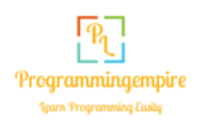