The following article demonstrates how to Display 2D Indexed Arrays in PHP.
- Example with numerical indices. The following code shows a 2D array with numeric indices.
$matrix = array(
array(1, 2, 3),
array(4, 5, 6),
array(7, 8, 9)
);
// Display the array
foreach ($matrix as $row) {
foreach ($row as $value) {
echo $value . " ";
}
echo "<br>";
}
The following output will be displayed.
1 2 3
4 5 6
7 8 9
- Example with string indices. The following example shows a 2D array with string indices.
$table = array(
array("name" => "John", "age" => 25, "city" => "New York"),
array("name" => "Mary", "age" => 30, "city" => "London"),
array("name" => "Bob", "age" => 20, "city" => "Paris")
);
// Display the array
foreach ($table as $row) {
foreach ($row as $key => $value) {
echo $key . ": " . $value . " ";
}
echo "<br>";
}
The following output will be generated.
name: John age: 25 city: New York
name: Mary age: 30 city: London
name: Bob age: 20 city: Paris
- Example with mixed indices. Likewise, we can have mixed indices.
$items = array(
array("item_id" => 1, "name" => "Apple", "price" => 1.5),
array("item_id" => 2, "name" => "Banana", "price" => 2.0),
array("item_id" => 3, "name" => "Orange", "price" => 1.0)
);
// Display the array
foreach ($items as $row) {
foreach ($row as $key => $value) {
echo $key . ": " . $value . " ";
}
echo "<br>";
}
The following output shows the mixed indices.
item_id: 1 name: Apple price: 1.5
item_id: 2 name: Banana price: 2
item_id: 3 name: Orange price: 1
- Example with dynamic indices. Similarly, we can have dynamic indices as shown below.
$grades = array();
$grades[] = array(80, 90, 70);
$grades[] = array(75, 85, 95);
$grades[] = array(60, 65, 70);
// Display the array
foreach ($grades as $row) {
foreach ($row as $value) {
echo $value . " ";
}
echo "<br>";
}
The following output will be shown.
80 90 70
75 85 95
60 65 70
- Example with the empty 2D array. In fact, we can have an empty @D array.
$empty_array = array(
array(),
array(),
array()
);
// Display the array
foreach ($empty_array as $row) {
foreach ($row as $value) {
echo $value . " ";
}
echo "<br>";
}
This will be the output.
<br>
<br>
<br>
Basically, in all these examples, I used nested loops to iterate over each row and column in the 2D array. Also, I have used the echo
statement to output the values in a readable format. Moreover, You can customize the output by using any HTML tags or formatting within the echo
statement.
Further Reading
Examples of Array Functions in PHP
Exploring PHP Arrays: Tips and Tricks
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
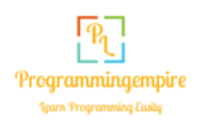