This article describes Different Methods of a Servlet.
Servlets are Java classes that extend the javax.servlet.http.HttpServlet
class and are primarily used to handle HTTP requests and responses. There are several HTTP methods (also known as HTTP verbs) that servlets can handle. The most common methods include the following.
- doGet(): This method is used to handle HTTP GET requests. It is typically used for reading or retrieving data from the server. The doGet() method is often used to implement read-only operations that do not modify server data.
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// Handling GET requests
}
- doPost(): This method is used to handle HTTP POST requests. It is typically used for submitting data to the server for processing or modification. The doPost() method is often used for operations that involve creating, updating, or deleting data on the server.
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// Handling POST requests
}
- doPut(): This method is used to handle HTTP PUT requests. It is typically used for updating data on the server. It is less commonly used in web applications compared to GET and POST.
@Override
protected void doPut(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// Handling PUT requests
}
- doDelete(): This method is used to handle HTTP DELETE requests. It is typically used for deleting data on the server. Like doPut(), it is less commonly used than doGet() and doPost().
@Override
protected void doDelete(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// Handling DELETE requests
}
- doHead(): This method is used to handle HTTP HEAD requests. A HEAD request is similar to a GET request, but it only requests the headers of the response, not the actual content. It is often used to check the availability and metadata of a resource without downloading the full content.
@Override
protected void doHead(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// Handling HEAD requests
}
- doOptions(): This method is used to handle HTTP OPTIONS requests. An OPTIONS request is used to retrieve information about the communication options for the target resource. It can be used to inquire about supported methods, headers, and other capabilities of the server.
@Override
protected void doOptions(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// Handling OPTIONS requests
}
- doTrace(): This method is used to handle HTTP TRACE requests. TRACE requests are used for diagnostic purposes and can be used to retrieve diagnostic information about the request-response cycle. It is less commonly used in practice.
@Override
protected void doTrace(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// Handling TRACE requests
}
In a typical servlet, you can override one or more of these methods to provide custom logic for handling different types of HTTP requests. The choice of which method to override depends on the specific functionality your servlet needs to implement.
Further Reading
Spring Framework Practice Problems and Their Solutions
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
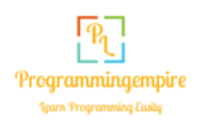