The following article describes the Difference Between super and this keyword in Java.
For the purpose of referring specific instances, we use super
and this
keywords in Java in a class hierarchy. As a matter of fact, the super
keyword refers to the parent class of the current class. Meanwhile, we can also use it to access members of the parent class that have been overridden by members of the current class. For example, if a subclass defines a method with the same name as a method in its parent class, we can use the super
keyword to call the parent class’s implementation of the method. The following code example demonstrates the use of the super
keyword to call a parent class’s method.
class Animal {
public void move() {
System.out.println("Animals can move");
}
}
class Dog extends Animal {
public void move() {
super.move();
System.out.println("Dogs can walk and run");
}
}
In this example, the Dog
class extends the Animal
class and overrides its move
method. When the Dog
class’s move
method is called, it first calls the move
method of the parent class (Animal
) using the super
keyword, and then adds its own behavior. The output of this code will be:
Animals can move
Dogs can walk and run
On the other hand, we use the this
keyword, to refer to the current instance of an object. Also, we can use it to access members of the current class, or to call other constructors in the same class. The following code example demonstrates how to use the this
keyword to call another constructor in the same class.
class Rectangle {
private int length;
private int width;
public Rectangle(int length, int width) {
this.length = length;
this.width = width;
}
public Rectangle(int side) {
this(side, side);
}
}
In this example, the Rectangle
class has two constructors. The second constructor takes a single argument side
, and calls the first constructor using the this
keyword, passing the same value for both the length
and width
arguments. This allows for creating a square rectangle by passing a single value for its side length.
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
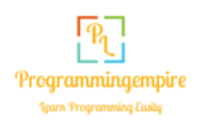